Workaround NotAllowedError in Safari
Problem: Calling HTML5 video play() programmatically gives NotAllowedError in Safari, even with muted set on the video element. This is often a problem when trying to load some video data asynchronously before calling play() , even though the playback was initally triggered by a user's click (which is required by Safari).
A workaround is to create an additional dummy video element, synchronously, when the user clicks the button, and then play it back immediately. Once that is done, the page is "whitelisted" by Safari and any other <video> tag can call play programmatically:

Can't play video on iOS in Safari: NotAllowedError: The request is not allowed by the user agent or the platform in the current context
When I click a play button on an iPhone in Safari, I get the following error:
NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission.
I searched on the internet and it seems that this issue has been around for a long time but there is no clear solution to it.
Here is my code. It works everywhere except iOS Safari.
import { Box } from '@chakra-ui/react'; import { useTranslation } from 'libs/i18next'; import React, { useEffect, useRef, useState } from 'react'; import { PauseIcon, PlayIcon } from 'theme/icons'; import { visuallyHiddenCss } from 'utils/style-utils'; import { buttonHoverArea, playButtonCss, playIconStyles, videoContainerCss, videoCss, } from './Video.styles'; export interface Video extends React.HTMLProps<HTMLVideoElement> { src: string; noVideoText?: string; className?: string; isEnabled?: boolean; coversParent?: boolean; handlePlayPress?: (videoPlaying: boolean) => void; } /** * Default HTML5 video player with a play button and a preview thumbnail image. */ export const Video = ({ src, noVideoText, className, loop = false, autoPlay = false, // AutoPlay doesn't work currently isEnabled = true, coversParent = false, handlePlayPress, ...props }: Video): React.ReactElement => { const [t] = useTranslation(['common']); const videoRef = useRef<HTMLVideoElement>(null); const buttonRef = useRef<HTMLButtonElement>(null); // use videoStarted state to hide the play button once it has been started const [videoPlaying, setVideoPlaying] = useState<boolean>(false); const pauseVideo = () => { setVideoPlaying(false); }; const toggleVideo = () => { if (handlePlayPress) { handlePlayPress(!videoPlaying); } setVideoPlaying(!videoPlaying); }; const defaultProps: Partial<Video> = { width: '100%', height: '100%', preload: 'none', onClick: pauseVideo, tabIndex: -1, }; const videoProps = Object.assign(defaultProps, props); useEffect(() => { if (videoRef.current && props.muted) { // force muted prop // https://github.com/facebook/react/issues/10389#issuecomment-605689475 videoRef.current.setAttribute('muted', ''); videoRef.current.defaultMuted = true; } }, [props.muted, videoRef]); useEffect(() => { if (isEnabled && videoPlaying) { videoRef.current?.play(); buttonRef.current?.blur(); videoRef.current?.focus(); } else { videoRef.current?.pause(); } }, [isEnabled, videoPlaying, videoRef]); const PlayPauseIcon = videoPlaying ? PauseIcon : PlayIcon; return ( <Box css={[ videoContainerCss.base, coversParent ? videoContainerCss.cover : undefined, ]} className={className} > {/* eslint-disable-next-line jsx-a11y/media-has-caption */} <video ref={videoRef} css={videoCss} {...videoProps} autoPlay={autoPlay} loop={loop} > <source src={src} type={'video/mp4'} /> {noVideoText} </video> {isEnabled && ( <div css={[buttonHoverArea.base, videoPlaying && buttonHoverArea.playing]} > <button type=button ref={buttonRef} css={playButtonCss} onClick={toggleVideo} // prevent hidden elements from being focused > <span css={visuallyHiddenCss}> {videoPlaying ? t('common:video.pause') : t('common:video.play')} </span> <PlayPauseIcon css={!videoPlaying && playIconStyles} boxSize={'6rem'} aria-hidden /> </button> </div> )} </Box> ); };
How can I avoid this error? And why is it happening?
Answers
The solution was to add muted property to the component which will be passed down to the video. Unfortunately it resulted in video being muted. In my case, I had <HomePage /> component and I passed muted down to the <Video /> component.
Possible solutions: add controllers or bind sound onto the play button (the latter might be not the best one).

kalaveronicab
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
How to fix NotAllowedError error when I try to play video in Safari browser?
I have created an application that uses Azure Media Player and Angular framework. I have a problem with playing the video in the Safari browser (OSX). If I try to play a video that is NOT muted, I got the following error in the Safari console:
Unhandled Promise Rejection: NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission.
I assumed that this error occurs when the app is trying to access the user's microphone, so I tried to prompt for microphone permission using the following code:
But it doesn't work, I am getting the same error. Please, do you what could be a potential solution for this problem?
Azure Media Services A group of Azure services that includes encoding, format conversion, on-demand streaming, content protection, and live streaming services. 305 questions Sign in to follow
Thanks for reaching here! Could you please elaborate your Safari version, Check this thread: https://github.com/scottschiller/SoundManager2/issues/178
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
HTMLMediaElement: play() method
The HTMLMediaElement play() method attempts to begin playback of the media. It returns a Promise which is resolved when playback has been successfully started.
Failure to begin playback for any reason, such as permission issues, result in the promise being rejected.
Return value
A Promise which is resolved when playback has been started, or is rejected if for any reason playback cannot be started.
Note: Browsers released before 2019 may not return a value from play() .
The promise's rejection handler is called with a DOMException object passed in as its sole input parameter (as opposed to a traditional exception being thrown). Possible errors include:
Provided if the user agent (browser) or operating system doesn't allow playback of media in the current context or situation. The browser may require the user to explicitly start media playback by clicking a "play" button, for example because of a Permissions Policy .
Provided if the media source (which may be specified as a MediaStream , MediaSource , Blob , or File , for example) doesn't represent a supported media format.
Other exceptions may be reported, depending on browser implementation details, media player implementation, and so forth.
Usage notes
Although the term "autoplay" is usually thought of as referring to pages that immediately begin playing media upon being loaded, web browsers' autoplay policies also apply to any script-initiated playback of media, including calls to play() .
If the user agent is configured not to allow automatic or script-initiated playback of media, calling play() will cause the returned promise to be immediately rejected with a NotAllowedError . Websites should be prepared to handle this situation. For example, a site should not present a user interface that assumes playback has begun automatically, but should instead update their UI based on whether the returned promise is fulfilled or rejected. See the example below for more information.
Note: The play() method may cause the user to be asked to grant permission to play the media, resulting in a possible delay before the returned promise is resolved. Be sure your code doesn't expect an immediate response.
For even more in-depth information about autoplay and autoplay blocking, see our article Autoplay guide for media and Web Audio APIs .
This example demonstrates how to confirm that playback has begun and how to gracefully handle blocked automatic playback:
In this example, playback of video is toggled off and on by the async playVideo() function. It tries to play the video, and if successful sets the class name of the playButton element to "playing" . If playback fails to start, the playButton element's class is cleared, restoring its default appearance. This ensures that the play button matches the actual state of playback by watching for the resolution or rejection of the Promise returned by play() .
When this example is executed, it begins by collecting references to the <video> element as well as the <button> used to toggle playback on and off. It then sets up an event handler for the click event on the play toggle button and attempts to automatically begin playback by calling playVideo() .
You can try out or remix this example in real time on Glitch .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Web media technologies
- Learning: Video and audio content
- Autoplay guide for media and Web Audio APIs
- Using the Web Audio API
Safari video not work - NotAllowedError:The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission

I ran into this error when I was pilot testing my online experiment using Safari. I didn’t have any issue when I ran the experiment using Chrome. I’m using macOS Ventura 13.1. My psychopy version is 2022.2.5. My safari is version 16.2.
I searched the forum, and some posts suggested that this error would be solved by setting the video to not auto-start. However, in my experiment, I created a play button and a pause button. The video does not auto-start. It actually starts to play after participant click the play button on the screen. And I’m still having this error in Safari (but not in Chrome). I have no idea what went wrong. Can someone please help with this? Thanks!!
Related Topics
Safari 11: audio.play causes "Unhandled Promise Rejection: NotAllowedError (DOM Exception 35)" even with user interaction.
Upon user interaction, I load an mp3 and when that completes (load event callback), I call audio.play(). However, I receive the following error Unhandled Promise Rejection: NotAllowedError (DOM Exception 35). I believe it has to do with calling audio.play() in the callback event. Is there a work around?
So I think technically the problem is because when a user clicks a play button, I make an async http call to get the download URL for that MP3. Because of the asynchronous nature, I have to load and play the mp3 in the subscription/success of that download URL http call. Perhaps Safari won't allow that since it's called on a separate, background thread.
Hi drall82, This is due to auto-play blocking for media with sound. The user gesture is not recognized by the element because of the delay caused by the async load ahead of play method call. It is likely it will work if you change the per-site auto-play preferences to always allow playing media with sound. If I recall, this is possible if you set the src attribute to trigger the load and call play() immediately. The element should already be created and attached to the DOM with no initial src attribute. Then on click you can set the src and call play() immediately. The media will play as soon as enough data has loaded. Maybe give that a shot and let me know if it works for you.
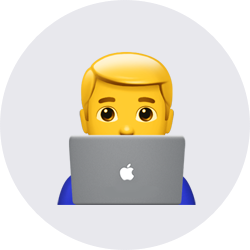
- Case Studies
- Support & Services
- Asset Store

Search Unity

A Unity ID allows you to buy and/or subscribe to Unity products and services, shop in the Asset Store and participate in the Unity community.
- Discussions
- Evangelists
- User Groups
- Beta Program
- Advisory Panel
You are using an out of date browser. It may not display this or other websites correctly. You should upgrade or use an alternative browser .
- If you have experience with import & exporting custom (.unitypackage) packages, please help complete a survey (open until May 15, 2024). Dismiss Notice
- Unity 6 Preview is now available. To find out what's new, have a look at our Unity 6 Preview blog post . Dismiss Notice
- Search titles only
Separate names with a comma.
- Search this thread only
- Display results as threads
Useful Searches
- Recent Posts
Video WebGL Safari videos will not play
Discussion in ' Audio & Video ' started by stephen-donohue , Feb 25, 2019 .
- videoplayer
stephen-donohue

I am trying to use the video player for a WebGL project. Videos will play fine on Chrome and Firefox, but on Safari I receive the following error. Code (JavaScript): Unhandled Promise Rejection : NotAllowedError : The request is not allowed by the user agent or the platform in the current context , possibly because the user denied permission. The videos are not set to auto-play, user must press a play button. If a user changes their auto-play option for the page to not "Stop media with sound" the video will play, however asking our users to change their settings is not an option, and regardless the media is not auto-playing. Our workaround in the past has been to split the audio into a separate mp3 file that we also load and sync to the video, but this is not an ideal solution. Is there a simpler workaround to get videos working properly in Safari?
AlexHJohnstone

Did you ever find a solution to this? Seems still unfixed as of 2019.1.10. I'm going to submit a bug report if there's no work-around.

I'm having troubles with Unity Video Player for years now... this system is really a problem to our app. We have a similar problem. Unity doesn't even give any information about that issue.

Any updates/fixes here?

apparently not

unity_aAWUp6wVMMsV-w

I still got problem to load url for video in videoplayer unity in safari and ios chrome.
kartoonist435

Having the same issue, have tried everything I can think of though mine works in every browser except Safari.

I was able to make it work in Safari. Have a look at my response here

GilCat said: ↑ I was able to make it work in Safari. Have a look at my response here Click to expand...

I made an asset to play videos on webgl, it works on Safari as well: https://assetstore.unity.com/packages/tools/video/videoplayerwebgl-192420

stephen-donohue said: ↑ I am trying to use the video player for a WebGL project. Videos will play fine on Chrome and Firefox, but on Safari I receive the following error. Code (JavaScript): Unhandled Promise Rejection : NotAllowedError : The request is not allowed by the user agent or the platform in the current context , possibly because the user denied permission. The videos are not set to auto-play, user must press a play button. Paris shuttle airport transfers prices If a user changes their auto-play option for the page to not "Stop media with sound" the video will play, however asking our users to change their settings is not an option, and regardless the media is not auto-playing. Our workaround in the past has been to split the audio into a separate mp3 file that we also load and sync to the video, but this is not an ideal solution. Is there a simpler workaround to get videos working properly in Safari? Click to expand...
matheus_inmotionvr

Any news on this? Anyone? Hello?

Marks4 said: ↑ I made an asset to play videos on webgl, it works on Safari as well: https://assetstore.unity.com/packages/tools/video/videoplayerwebgl-192420 Click to expand...
@Ikaro88 No, but I guarantee it works. Please see the vid and reviews. If you run into any problems, don't hesitate to contact me!
213277/can-t-play-video-on-ios-in-safari-notallowederror
- Mobile Development
Can t play video on iOS in Safari NotAllowedError
When I click a play button on an iPhone in Safari, I get the following error:
NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission.
I searched on the internet and it seems that this issue has been around for a long time but there is no clear solution to it.
Here is my code. It works everywhere except iOS Safari.
- html5-video
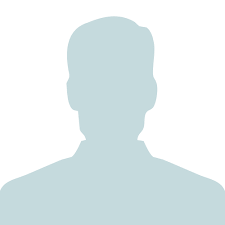
Your comment on this question:
No answer to this question. be the first to respond., your answer, related questions in mobile development, open webpage in fullscreen in safari on ios.
Not all platforms are equal. iOS Safari doesn't ... READ MORE
- mobile-safari
Default Ringtone on a VOIP app in iOS
I'm trying to find a way to ... READ MORE
Flutter project in iOS emulator takes forever to run, but its worked on Android emulator
Try flutter clean and flutter build ios ... READ MORE
- android-emulator

Twilio caller name on receive programmable voice call in ios application
Twilio developer evangelist here. In order to get ... READ MORE
- twilio-programmable-voice
Streaming video over WiFi and Bluetooth on iOS
This question is going to be quite ... READ MORE
- video-streaming
Error:Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag
Hello @kartik, It is happening because any where ... READ MORE
Error:setState doesn't update the state immediately
Hello @kartik, The method setState() takes a callback. And ... READ MORE
Error:React.createElement: type is invalid — expected a string (for built-in components) or a class/function (for composite components) but got: object
The issue you have encountered was caused ... READ MORE
From php returning JSON to JavaScript
Hii @kartik, You can use Simple JSON for PHP. ... READ MORE
- All categories
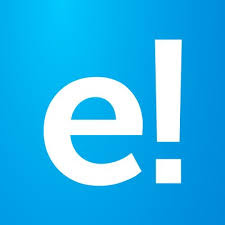
Join the world's most active Tech Community!
Welcome back to the world's most active tech community.
At least 1 upper-case and 1 lower-case letter
Minimum 8 characters and Maximum 50 characters
Subscribe to our Newsletter, and get personalized recommendations.

Already have an account? Sign in .
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Can't auto-play video from a flutter web app #68482
wer-mathurin commented Oct 19, 2020 • edited by TahaTesser
TahaTesser commented Oct 19, 2020
Sorry, something went wrong.
wer-mathurin commented Oct 19, 2020
MichealReed commented Oct 19, 2020
- 👍 2 reactions
github-actions bot commented Aug 11, 2021
No branches or pull requests
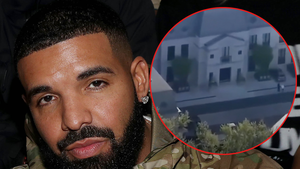
Drake's Toronto Home Visited By Yet Another Alleged Attempted Trespasser
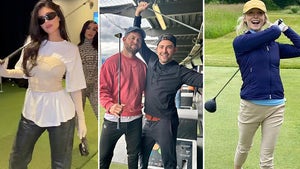
See Which Stars Are Swinging Their Clubs To Tee Off Golf Day!
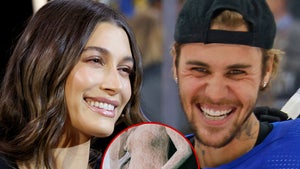
Hailey Bieber Pregnant with Justin Bieber's Baby, Six Months Along
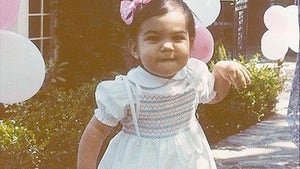
Guess Who This Dressed Up Cutie Turned Into!
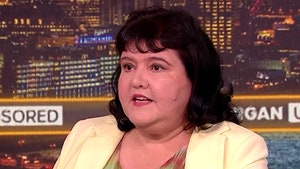
Real-Life 'Baby Reindeer' Martha Says She Sent Gadd Less Than 10 Emails
Lions have sex on top of safari truck full of people, wild video, roaring sex lions get busy on top of safari truck ... tourists take it all in.
A couple of lions felt the love so much that they started banging on top of a tourist safari truck ... and the people inside certainly got an eyeful and then some.
A group of tourists partaking in a South African safari were recently left stunned as 2 randy big cats made themselves at home atop their vehicle.
The male lion is seen mounting a resting female lion ... confirming their boinking session with a couple of growls. The jeep noticeably shakes from side to side as they do it ... sparking laughter and gasps from the tourists seated below.
The lions' intimacy didn't last too long ... with the lioness lying tired to the side as the king of the jungle eventually disconnected.
While the encounter may have given some tourists the ick on the spot ... it's significantly less scary than the African elephant attack from earlier this month -- which ended in a death.
No one died here ... just a couple of kitties getting laid!
- Share on Facebook
related articles
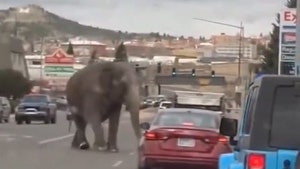
Elephant Roams Montana Streets After Escaping Circus
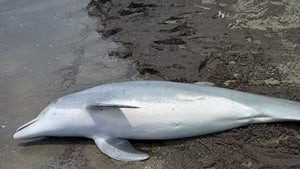
Dolphin That Washed Ashore in Louisiana Was Shot & Killed, Officials Say
Old news is old news be first.

IMAGES
VIDEO
COMMENTS
The solution was to add muted property to the component which will be passed down to the video. Unfortunately it resulted in video being muted. In my case, I had <HomePage /> component and I passed muted down to the <Video /> component. Possible solutions: add controllers or bind sound onto the play button (the latter might be not the best one).
using peer.js for stream video on React APP addVideoStream(video: HTMLVideoElement, stream: MediaStream) { video.srcObject = stream video?.addEventListener ...
The video is muted. Once I click anywhere inside the body the play no longer gets blocked. notallowederror: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. The behaviour is the same when I select the default option "Stop Media with Audio".
This is often a problem when trying to load some video data asynchronously before calling play(), even though the playback was initally triggered by a user's click (which is required by Safari). A workaround is to create an additional dummy video element, synchronously, when the user clicks the button, and then play it back immediately.
When I click a play button on an iPhone in Safari, I get the following error: NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. I searched on the internet and it seems that this issue has been around for a long time but there is no clear solution to it.
Interesting, I don't think I've seen this before in Safari. That said, these Unhandled Promise Rejections are usually harmless and can be ignored. It does seem that seeking a lot causes it, so maybe we could make sure we silence the play promise anywhere we call play() in Video.js.
A group of Azure services that includes encoding, format conversion, on-demand streaming, content protection, and live streaming services.
In this example, playback of video is toggled off and on by the async playVideo() function. It tries to play the video, and if successful sets the class name of the playButton element to "playing".If playback fails to start, the playButton element's class is cleared, restoring its default appearance. This ensures that the play button matches the actual state of playback by watching for the ...
The video does not auto-start. It actually starts to play after partic... psychopy.org | Reference | Downloads | Github. PsychoPy Safari video not work - NotAllowedError:The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. Online experiments.
I setup a simple scene with a button and a video player that load a video hosted on Digital Ocean. The video works well everywhere but it doesn't load on iOS, i see only a black screen. I read through the internet that can also be a CORS problem (which i setup on DO). Do you have any idea of what is wrong? Here the CORS settings. Thank you in ...
Hi drall82, This is due to auto-play blocking for media with sound. The user gesture is not recognized by the element because of the delay caused by the async load ahead of play method call. It is likely it will work if you change the per-site auto-play preferences to always allow playing media with sound. If I recall, this is possible if you ...
The videos are not set to auto-play, user must press a play button. Paris shuttle airport transfers prices If a user changes their auto-play option for the page to not "Stop media with sound" the video will play, however asking our users to change their settings is not an option, and regardless the media is not auto-playing.
When I click a play button on an iPhone in Safari, I get the following error: NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. I searched on the internet and it seems that this issue has been around for a long time but there is no clear solution to it.
edited by TahaTesser. Steps to Reproduce. Use the example plugin from video_player plugins. Since mixWithOthers is not implemented on web. Just remove the video player option from the main.dart in the initState method. so comment or remove this line. videoPlayerOptions: VideoPlayerOptions(mixWithOthers: true),
A couple of lions felt the love so much that they started banging on top of a tourist safari truck ... and the people inside certainly got an eyeful and then some.
If I change the autoplay setting in safari to 'Allow all Autoplay', video is playing fine with out any issues. I tried by adding autoplay attribute also to Video element but it is not working. Expectation is user shouldn't do any thing to play the video.
Since the last Safari update to version 11, some videos stopped working. The worst is that sometimes, like once in five attempts, it works. I thought that the reason is my JS script that integrates Vimeo Player API but it ends up that even Vimeo's embed link doesn't work at all.
If the video is not working in Safari, but works in other browsers (Chrome, Firefox, Edge), you may be running into an issue relating to byte-range requests. Byte-range requests are when a client asks a server for only a specific portion of the requested file. The primary purpose of this is to conserve bandwidth usage by only downloading small ...
2. I'm struggling to play an audio (mp3 file) on Safari. It returns: [Error] Unhandled Promise Rejection: NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. The same is working just fine on Chrome. I understand that Safari won't allow autoplay if the ...
WebKit brings the Async Clipboard API to this release of Safari... you should read it. TLDR: The implementation is available through the navigator.clipboard API which must be called within user events. You are trying to copy to the clipboard as a result of an API call instead of in the context of a user interaction. This is not allowed.