Safari Web Content Guide
- Table of Contents
- Jump To
- Download Sample Code

Getting Geographic Locations
Use the JavaScript classes described in this chapter to obtain or track the current geographic location of the host device. These classes hide the implementation details of how the location information is obtained—for example, using Global Positioning System (GPS), IP addresses, Wi-Fi, Bluetooth, or some other technology. The classes allow you to get the current location or get continual updates on the location as it changes.
Geographic Location Classes
The Navigator object has a read-only Geolocation instance variable. You obtain location information from this Geolocation object. The parameters to the Geolocation methods that get location information are mostly callbacks, instances of PositionCallback or PositionErrorCallback . Because there may be a delay in getting location information, it cannot be returned immediately by these methods. The callbacks that you specify are invoked when the location information is obtained or an error occurs. If the location information is obtained, the position callback is passed a position object describing the geographic location. If an error occurs, the error callback is passed an instance of PositionError describing the error. The position object represents the location in latitude and longitude coordinates.
Getting the Current Location
The most common use of the Geolocation class is to get the current location. For example, your web application can get the current location and display it on a map for the user. Use the getCurrentPosition method in Geolocation to get the current location from the Navigator object. Pass your callback function as the parameter to the getCurrentPosition method as follows:
Your callback function—the showMap function in this example—should take a position object as the parameter as follows:
Use the coords instance variable of the passed-in position object to obtain the latitude and longitude coordinates as follows:
Tracking the Current Location
You can also track the current location. For example, if your web application displays the current location on a map, you can register for location changes and continually scroll the map as the current location changes. When you register for location changes, you receive a callback every time the location changes. The callbacks are continual until you unregister for location changes.
Use the watchPosition method in the Geolocation class to register for location changes. Pass your callback function as the parameter. In this example, the scrollMap function is invoked every time the current location changes:
The callback function should take a position object as the parameter as follows:
Similar to Getting the Current Location , use the coords instance variable of the passed in position object to obtain the latitude and longitude coordinates.
Use the clearWatch method in the Geolocation class to unregister for location changes. For example, unregister when the user clicks a button or taps a finger on the map as follows:
Handling Location Errors
Your web application should handle errors that can occur when requesting location information. For example, display a message to the user if the location cannot be determined due to poor network connectivity or some other error.
When registering for location changes, you can optionally pass an error callback to the watchPosition method in the Geolocation class as follows:
The error callback should take a PositionError object as the parameter as in:
Copyright © 2016 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2016-12-12
Sending feedback…
We’re sorry, an error has occurred..
Please try submitting your feedback later.
Thank you for providing feedback!
Your input helps improve our developer documentation.
How helpful is this document?
How can we improve this document.
* Required information
To submit a product bug or enhancement request, please visit the Bug Reporter page.
Please read Apple's Unsolicited Idea Submission Policy before you send us your feedback.
How to use the Geolocation API
New Courses Coming Soon
Join the waiting lists
Using the Geolocation API we can ask the browser for the user's position coordinates
The browser exposes a navigator.geolocation object, through which we’ll do all the geolocation operations.
It’s only available on pages served using HTTPS, for security purposes, and it’s available on all modern browsers.
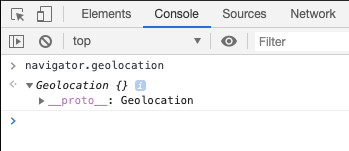
Since window is the global object, we can access navigator without specifying window.navigator
The window.navigator property exposed by browsers points to a Navigator object which is a container object that makes a lot of Web Platform APIs available to us.
The geolocation object provides the following methods:
- getCurrentPosition()
- watchPosition()
- clearWatch()
The first one is used to get the current position coordinates. When we call this method for the first time, the browser automatically asks the user for the permission to share this information to us.
This is how this interface looks in Chrome:
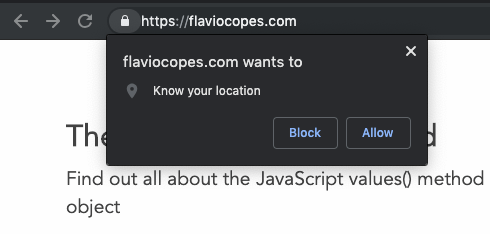
on Firefox:
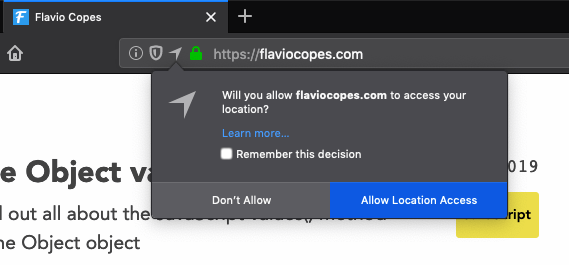
and on Safari:
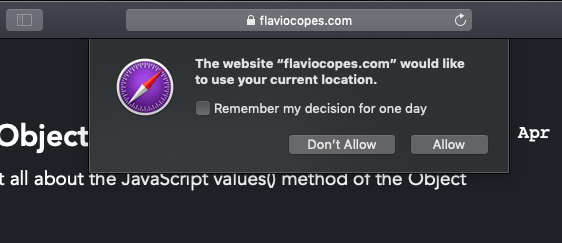
This needs to be done only once per origin. You can change the decision you made, and revert a permission decision. This is how you do so with Chrome, by clicking the lock icon near the domain:
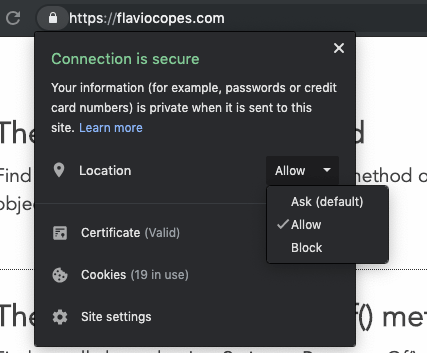
Once this permission is granted, we can proceed.
Getting the user’s position
Let’s start with this sample code:
The permission panel should appear. Allow the permission.
Notice how I passed an empty arrow function , because the function wants a callback function.
This function is passed a Position object, which contains the actual location:
This object has 2 properties:
- coords , a Coordinates object
- timestamp , the UNIX timestamp when the position was retrieved
The Coordinates object comes with several properties that define the location:
- accuracy the accuracy of the position measured, expressed in meters
- altitude the altitude value measured
- altitudeAccuracy the accuracy of the altitude measured, expressed in meters
- heading the direction towards which the device is traveling. Expressed in degrees (0 = North, East = 90, South = 180, West = 270)
- latitude the latitude value measured
- longitude the longitude value measured
- speed the speed at which the device is traveling, expressed in meters per second
Depending on the implementation and the device, some of those will be null . For example on Chrome running on my MacBook Air I only got values for accuracy , latitude and longitude .
Watching the position for changes
In addition to getting the user position once, which you can do using getCurrentPosition() , you can use the watchPosition() method of navigator.geolocation to register a callback function that will be called upon each and every change that the device will communicate to us.
Of course the browser will ask for the permission also with this method, if it was not already granted.
We can stop watching for a position by calling the navigator.geolocation.clearWatch() method, passing it the id returned by watchPosition() :
If the user denies the permission
Remember the permission popup window the browser shows when we call one of the methods to get the position?
If the user denies that, we can intercept this scenario by adding an error handling function, as the second parameter to the methods getCurrentPosition() and watchPosition() .
The object passed to the second parameter contains a code property to distinguish between error types:
- 1 means permission denied
- 2 means position unavailable
- 3 means timeout
Adding more options
When I talked about errors previously, I mentioned the timeout error. Looking up the position can take some time and we can set a maximum time allowed to perform the operation, as an option.
You can add a timeout by adding a third parameter to the methods getCurrentPosition() and watchPosition() , an object.
Inside this object we can pass the properties
- timeout to set the number of milliseconds before the request errors out
- maximumAge to set the maximum “age” of the position cached by the browser. We don’t accept one older than the set amount of milliseconds
- enableHighAccuracy a boolean by default false , requires a position with the highest level of accuracy possible (which might take more time and more power)
Example usage:
Here is how can I help you:
- COURSES where I teach everything I know
- CODING BOOTCAMP cohort course - next edition in 2025
- BOOKS 16 coding ebooks you can download for free on JS Python C PHP and lots more
- Follow me on X
Back to all guides
Interactive Guide to Geolocation API
Mon Apr 18 2022

Having access to your user’s location can be quite valuable. If you embed this feature into your website, you can utilize your user’s location data and perform certain operations. For instance, you can provide appropriate discounts on your e-commerce store based on your user’s location. You can also show your users tailored content based on their current location.
There are different ways you can get the location of your customers. There are several paid APIs available to help you get the location of your customers. The Geolocation web API may help you. Let’s take a deeper look into this API.
Geolocation API
This web API returns the geographical latitudes and longitudes and the accuracy to which it is correct. It utilizes the device’s GPS chip to get location. If the chip is not present, it uses the cellular triangulation method to get the coordinates. The accuracy may vary in this case along with other factors.
The Geolocation API requires the user to explicitly permit the website to enable location services. The permission can later be overridden if the user desires.
The API provides three methods. Let’s briefly look at each of them.
getCurrentPosition()
As the name suggests, this method provides the user's current location data. It takes three parameters, among which two are optional.
The first is a callback function that will execute once the API has the location. It will take a single location object as a parameter. The second callback function is optional, but it is used to handle the error. The third parameter is also optional, and it is an object that defines options for the API.
watchPosition()
This method registers the device location and then executes the callback function whenever the position changes with respect to the original position.
clearWatch()
This method unregisters the effect of watchPosition() method.
Let’s look at how you can use this API to store credentials.
Create a directory on your computer and open it in your preferred code editor. Once you are done, create an HTML file called index.html inside this directory. Now write a basic HTML boilerplate. I have also provided it below that you can copy and paste.
Now create a button that will get the current user location. Ensure that you attach an onclick event handler to this button.
Now inside the script tag, write a getLocation function that will call the Geolocation API. In the end, the code will look like this:
Now run this file and click on the Get location button. Afterward, open the browser console. You will find out that the latitudes, longitudes, and accuracy have been logged there.
The Geolocation API is supported across all major internet browsers, including Chrome, Firefox, and Safari. Although, for security reasons, your site would need an SSL certificate to use this web API.
- Skip to main content
- Select language
- Skip to search
Using geolocation
Fine tuning response, live result.
The geolocation API allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information.
The geolocation object
The geolocation API is published through the navigator.geolocation object.
If the object exists, geolocation services are available. You can test for the presence of geolocation thusly:
Note: On Firefox 24 and older versions, "geolocation" in navigator always returned true even if the API was disabled. This has been fixed with Firefox 25 to comply with the spec. ( bug 884921 ).
Getting the current position
To obtain the user's current location, you can call the getCurrentPosition() method. This initiates an asynchronous request to detect the user's position, and queries the positioning hardware to get up-to-date information. When the position is determined, the defined callback function is executed. You can optionally provide a second callback function to be executed if an error occurs. A third, optional, parameter is an options object where you can set the maximum age of the position returned, the time to wait for a request, and if you want high accuracy for the position.
Note: By default, getCurrentPosition() tries to answer as fast as possible with a low accuracy result. It is useful if you need a quick answer regardless of the accuracy. Devices with a GPS, for example, can take a minute or more to get a GPS fix, so less accurate data (IP location or wifi) may be returned to getCurrentPosition() .
The above example will cause the do_something() function to execute when the location is obtained.
Watching the current position
If the position data changes (either by device movement or if more accurate geo information arrives), you can set up a callback function that is called with that updated position information. This is done using the watchPosition() function, which has the same input parameters as getCurrentPosition() . The callback function is called multiple times, allowing the browser to either update your location as you move, or provide a more accurate location as different techniques are used to geolocate you. The error callback function, which is optional just as it is for getCurrentPosition() , can be called repeatedly.
Note: You can use watchPosition() without an initial getCurrentPosition() call.
The watchPosition() method returns an ID number that can be used to uniquely identify the requested position watcher; you use this value in tandem with the clearWatch() method to stop watching the user's location.
Both getCurrentPosition() and watchPosition() accept a success callback, an optional error callback, and an optional PositionOptions object.
A call to watchPosition could look like:
A demo of watchPosition in use: http://www.thedotproduct.org/experiments/geo/
Describing a position
The user's location is described using a Position object referencing a Coordinates object.
Handling errors
The error callback function, if provided when calling getCurrentPosition() or watchPosition() , expects a PositionError object as its first parameter.
Geolocation Live Example
Html content, javascript content, prompting for permission.
Any add-on hosted on addons.mozilla.org which makes use of geolocation data must explicitly request permission before doing so. The following function will request permission in a manner similar to the automatic prompt displayed for web pages. The user's response will be saved in the preference specified by the pref parameter, if applicable. The function provided in the callback parameter will be called with a boolean value indicating the user's response. If true , the add-on may access geolocation data.
Browser compatibility
[1] Firefox includes support for locating you based on your WiFi information using Google Location Services. In the transaction between Firefox and Google, data is exchanged including WiFi Access Point data, an access token (similar to a 2 week cookie), and the user's IP address. For more information, please check out Mozilla's Privacy Policy and Google's Privacy Policy covering how this data can be used.
Firefox 3.6 (Gecko 1.9.2) added support for using the GPSD (GPS daemon) service for geolocation on Linux.
- navigator.geolocation
- Plotting yourself on the map
- Geolocation API on w3.org
- Who moved my geolocation? (Hacks blog)
Document Tags and Contributors
- Geolocation API
- Intermediate

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
HTML5 Geolocation in Safari 5
HTML5 Geolocation API lets you share your location with your favorite web sites. A JavaScript can capture your latitude and longitude, can be sent to backend web server, and do fancy location-aware things like finding local businesses or showing your location on a map.
Let us see how to get the current position −

Related Articles
- HTML5 geolocation 'permission denied' error in Mobile Safari
- Detect folders in Safari with HTML5
- HTML5 Geolocation without SSL connection
- How to use geolocation coordinates in HTML5?
- How to handle Geolocation errors in HTML5?
- Chrome and HTML5 GeoLocation denial callback
- HTML5 date field and placeholder text in Safari
- How to find position with HTML5 Geolocation?
- Geolocation HTML5 enableHighAccuracy True, False or What?
- Error codes returned in the PositionError object HTML5 Geolocation
- Raise the Mobile Safari HTML5 application cache limit?
- How to use HTML5 Geolocation Latitude/Longitude API?
- How to use HTML5 GeoLocation API with Google Maps?
- Getting Safari to recognize HTML 5
- How to best display HTML5 geolocation accuracy on a Google Map?
Kickstart Your Career
Get certified by completing the course
To Continue Learning Please Login
Geolocation
Method of informing a website of the user's geographical location
- 4 : Partial support
- 5 - 49 : Supported
- 50 - 124 : Supported
- 125 : Supported
- 126 - 128 : Supported
- 12 - 18 : Supported
- 79 - 124 : Supported
- 3.1 - 4 : Not supported (but has polyfill available)
- 5 - 9.1 : Supported
- 10 : Supported
- 10.1 - 17.4 : Supported
- 17.5 : Supported
- 17.6 - TP : Supported
- 2 - 3 : Not supported (but has polyfill available)
- 3.5 - 54 : Supported
- 55 - 125 : Supported
- 126 : Supported
- 127 - 129 : Supported
- 9 - 9.6 : Not supported
- 10 - 10.5 : Not supported (but has polyfill available)
- 10.6 - 12.1 : Supported
- 15 : Not supported
- 16 - 38 : Supported
- 39 - 109 : Supported
- 110 : Supported
- 5.5 : Not supported
- 6 - 8 : Not supported (but has polyfill available)
- 9 - 10 : Supported
- 11 : Supported
Chrome for Android
Safari on ios.
- 3.2 - 9.3 : Supported
- 10 - 17.4 : Supported
- 17.6 : Supported
Samsung Internet
- 4 : Supported
- 5 - 24 : Supported
- 25 : Supported
- all : Not supported
Opera Mobile
- 10 : Not supported (but has polyfill available)
- 11 - 12.1 : Supported
- 80 : Supported
UC Browser for Android
- 15.5 : Supported
Android Browser
- 2.1 - 4.4.4 : Supported
Firefox for Android
- 14.9 : Supported
Baidu Browser
- 13.52 : Supported
KaiOS Browser
- 2.5 : Supported
- 3 : Supported

Using the HTML Geolocation API to Add App-Like User Experience to your PWA
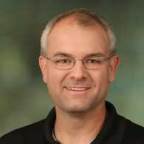
Last Updated - Sat Jan 09 2021
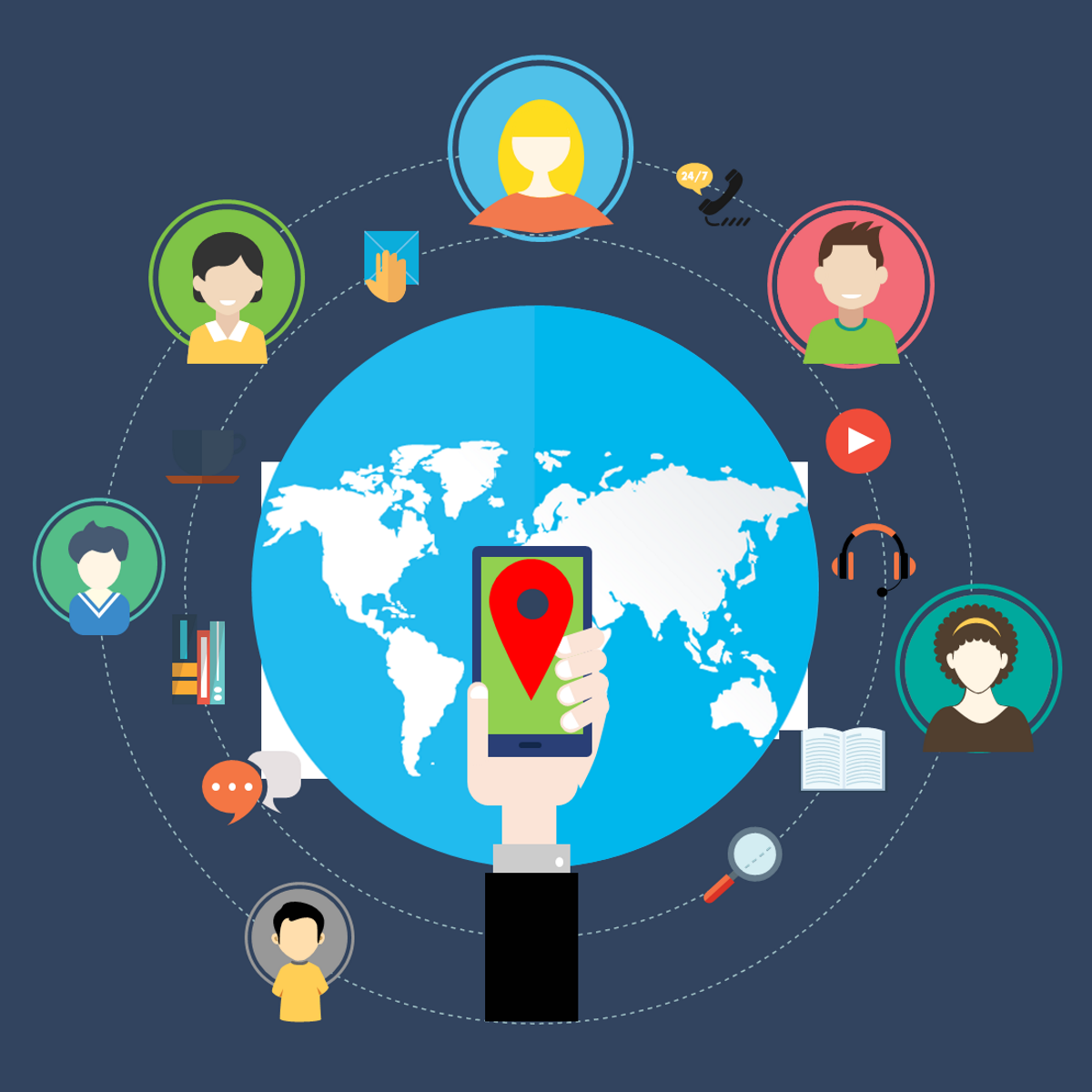
Geolocation is more important now than ever before. It is a great way to add an ‘app-like’ feature to your progressive web application . We like to know what business, attractions and destinations are around, where we are, and how to get where we are going.
Browsers offer a common JavaScript geolocation service you can use to enrich your HTML driven experiences.
A common use of geolocation is to show where stores are located and possible driving directions.
Geolocation is not limited to just retail. Delivery and driving services use location to update you and the drivers where they are and how much time remains. I use it to find places of interest relative to my location.
Now that Smartphones are ubiquitous, we want information right now, wherever we are. Popular applications like Google Maps, Yelp and Facebook let us locate businesses and friends near our locations right from our phone.
I have been fascinated with geolocation-based data for several years now and have watched the technology evolve. Before smartphones, determining a user's location was to limited to mapping through a geocoordinated IP database.
To access a geocoordinated database, you either needed to subscribe to a service which would translate an IP address to an approximate latitude and longitude, or you needed to maintain a local database with this information. This also needed to be done on the server, which adds latency.
Either option was not cheap or completely reliable. In my experience, an IP address does not actually correlate to a device's location all the time.
For example, I used to have a Sprint 3G service for my laptop. The account that activated my service was located in the Chicago, IL area. Whenever I visited sites using geolocated content, I would receive content related to the Chicago metro area.
The funny thing is the only time I was in the Chicago metro area was changing planes at the airport! We have had a better way to target a user's location thanks to the http://www.w3.org/TR/geolocation-API/” HTML GeoLocation API.
The HTML Geolocation API
All browsers support and have supported native support for the geolocation API for about a decade. So, the API is safe to use.
Using the geolocation API combined with a map service like Microsoft Bing Maps or Google Maps Platforms provide a rich end user experience. You can add a map service to your site to add more value and app like experiences to extend your brand.
Now you can pinpoint where the visitor is located and add nearby locations to a map, provide driving directions and more. You should be aware these services do charge for some features. You will need to consult their service pages to understand their pricing model so you won’t be surprised.
Even though every browser supports the Geolocation API it is still advisable to feature detect if the API is supported. The geolocation object should be a member of the navigator object, so you can check if it is in the navigator.
Detecting Geolocation Support
A reason you should feature detect is just in case the user has disabled the service. You should also be aware that modern browsers are now gating the API behind HTTPS and some require getting the user’s permission before the API works.
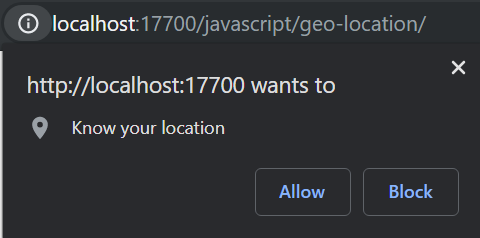
You can use HTTP when working locally, using the localhost origin. This helps facilitate development. The geolocation object has three methods:
- getCurrentPosition : a direct call to get the device’s current position
- watchPosition : triggers when the device’s location changes
- clearWatch : stops or clears the watch created by calling the watchPosition method
Using getCurrentPosition
The simplest use of the geolocation object is to get the device location in a single call using the getCurrentPosition method.
The method has three parameters, a success callback, an error callback and options object. The last two parameters are optional.
The success callback will have a single parameter passed, a Position object. Likewise, the error callback receives a single parameter, a PositionError object.
The options parameter is a PositionOptions object. It has three properties:

- maximumAge : an integer (milliseconds) indicating how old a cached position is valid
- timeout : how many milliseconds before the error handler is invoked, default is no timeout
- enableHighAccuracy : true or false, false by default. Enabling results in more power consumption and more time to collect position.
All PositionOptions properties are optional. If a value is not supplied the geolocation system uses defaults. The Position object passed to the success callback has two properties, coords (a Coordinates object) and timestamp. The coordinates have all the (read only) values we are after:
- latitude : double
- longitude : double
- altitude : double, meters above sea level
- accuracy : accuracy or radius of accuracy in meters
- altitudeAccuracy : accuracy or radius of accuracy in meters
- heading : how many degrees from true North the device is moving
- speed : velocity in meters/second the device is moving
You should note not all values will be supplied. You will always get at least the latitude and longitude. I will review accuracy a little later.
Altitude depends on the device capabilities, so you should account for the values not being provided. Create a graceful enhancement experience when the altitude add value to the application experience.
The timestamp value is a Date object specifying when the location was determined.
Handling Position Errors
If there was a problem capturing the user’s device position the error callback function will be triggered. The PositionError object contains a numeric Code property, and a message. The code property is an unsigned_short value you can match from the error object’s value.
- UNKNOWN_ERROR : Code 0, The most frustrating error because the position could not be determined and the browser does not know why
- PERMISSION_DENIED : Code 1, The user denied permission to use the geolocation API from the permission prompt
- POSITION_UNAVAILABLE : Code 2, The device location was not available
- TIMEOUT: Code 3, The position could not be collected within the timeout interval
This is an example of how a geolocation error handling callback might work.

Tracking Position Changes
The getCurrentPosition is great to get the user’s current location. But if your application needs to track the user’s position change, think turn by turn driving directions or tracking a run, having the device provide updated coordinates is more efficient.
Thankfully the geolocation watchPosition method triggers callbacks when the device location is updated. If it were not for this you would be responsible for using a setInterval or requestAnimationFrame method to call getCurrentPosition over and over.
Having the device trigger an update when the device update’s its position is more efficient. This preserves the battery and excessive CPU usage.
The watchPosition method has the same signature as getCurrentPosition, so you can easily update to use the watchPosition method.
The method returns a numeric id, similar to setInterval. You can use this id to later stop the watch.
The geolocation clearWatch method accepts the watch id and clears the watch callback. In this example I pass the geoWatch id to the clearWatch method to stop the callback. I further set the variable to undefined because I check if it is being used before calling the watchPosition method.
Now you can turn your phone into an expensive step counter!
Just for fun you could also create a progressive web application version of Pokemon Go. The geolocation watchPosition method can be used to track where the player is located so you can display little monsters. I will leave it up to you to figure out how to use the monsters for target practice.
Setting Coordinates Using the Chromium Developer Tools
In Chrome, new Edge and other Chromium based browsers the developers tools have a ‘sensors’ tab. In this panel you can set the device’s latitude and longitude. You can also adjust the device orientation, but that comes in handy with the gyroscope API.
The Geolocation sensor emulation comes with many major world cities already preset. You can add additional locations as needed.
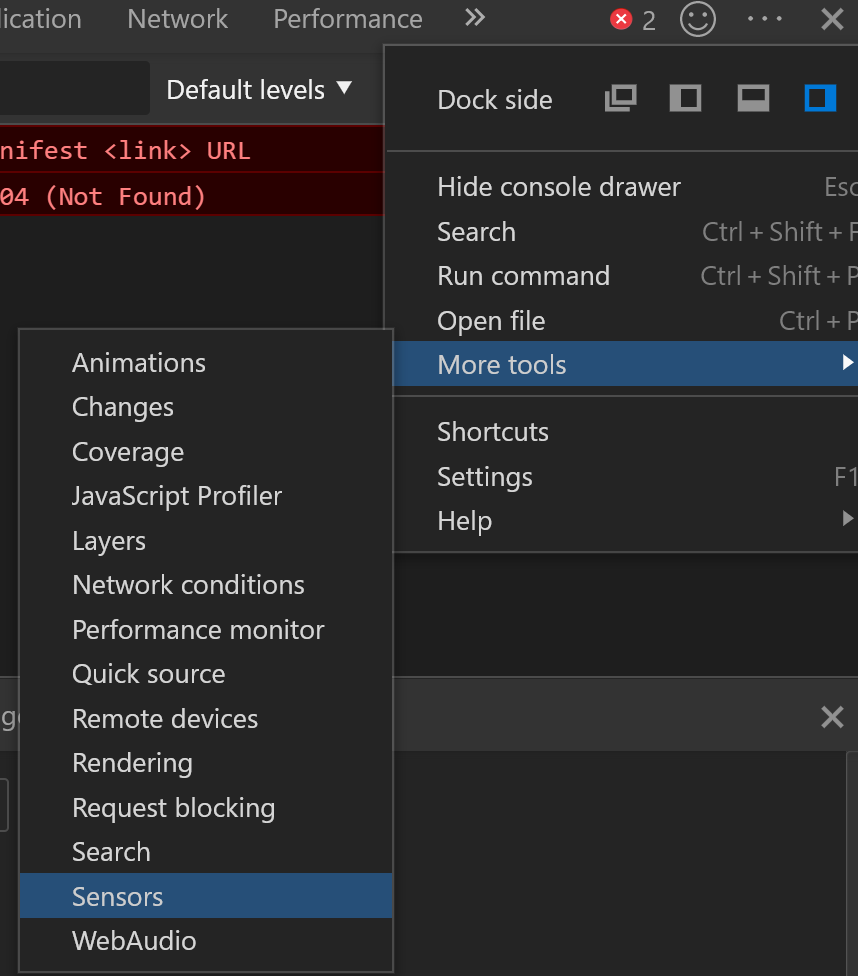
To select a location just press the input field to the right of ‘Geolocation’. It looks like a text input, but it will display a list of available locations.
Pressing the ‘Manage’ button will display a list of locations and the ability to add a new location.
This is a great feature to use when you are developing geolocation dependent applications. The only drawback is you can’t emulate a moving device. But since the watchPosition has the same signature as getCurrentPosition method you can at least make sure your workflow processes the position object correctly.
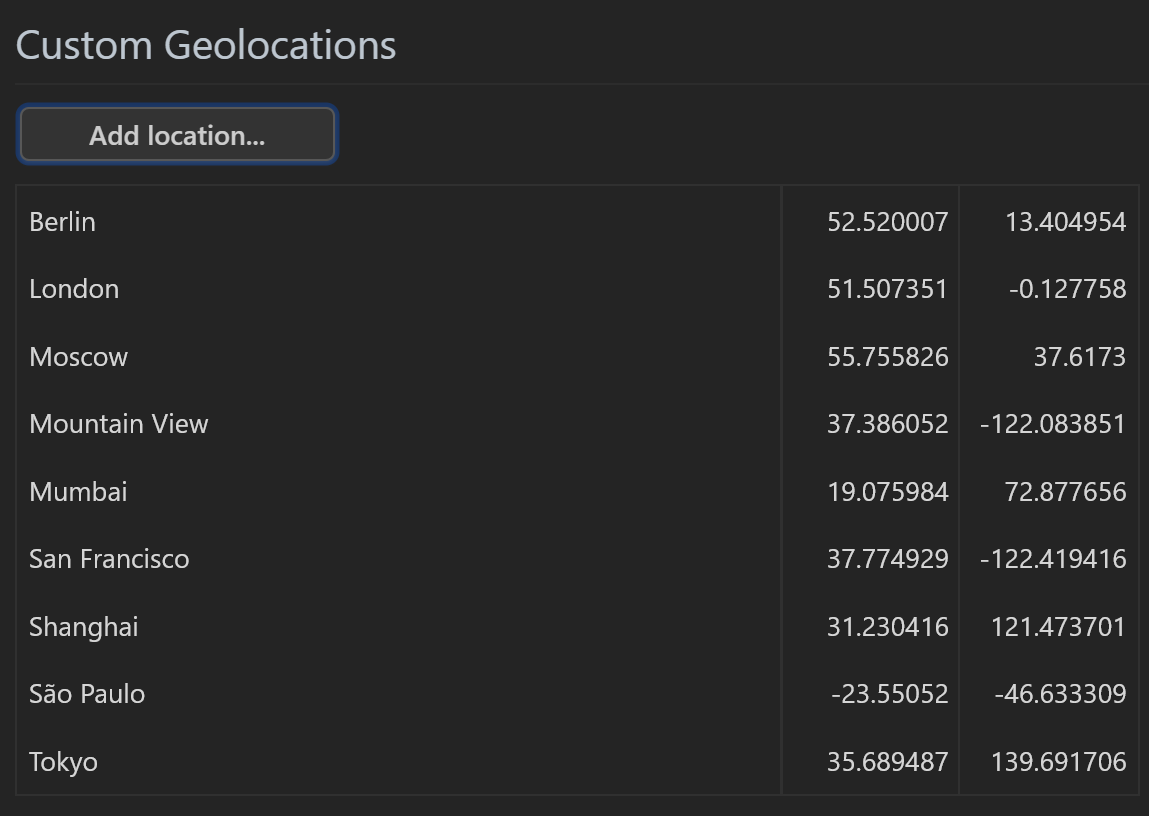
How Position is Determined
There are three ways devices determine location.
- Cell Tower Triangulation
When using Wifi the network router is normally a fixed location and turns out to be well known. There are several highly accurate services that track that sort of thing and all major platform vendors use one or more of these services to determine a devices position.
Remember I said in the old days you needed to have access to an IP database and the more accurate the database or service the more expensive it was? Well no more, Apple, Google, Microsoft, etc have taken care of this for you, so long as you use the geolocation API.
In my experience these values usually have my location pinned to within 100 feet or about 30 meters. Browsers are not the determining factor when determining the location and accuracy. They simply query the operating system’s interface to the GPS hardware.
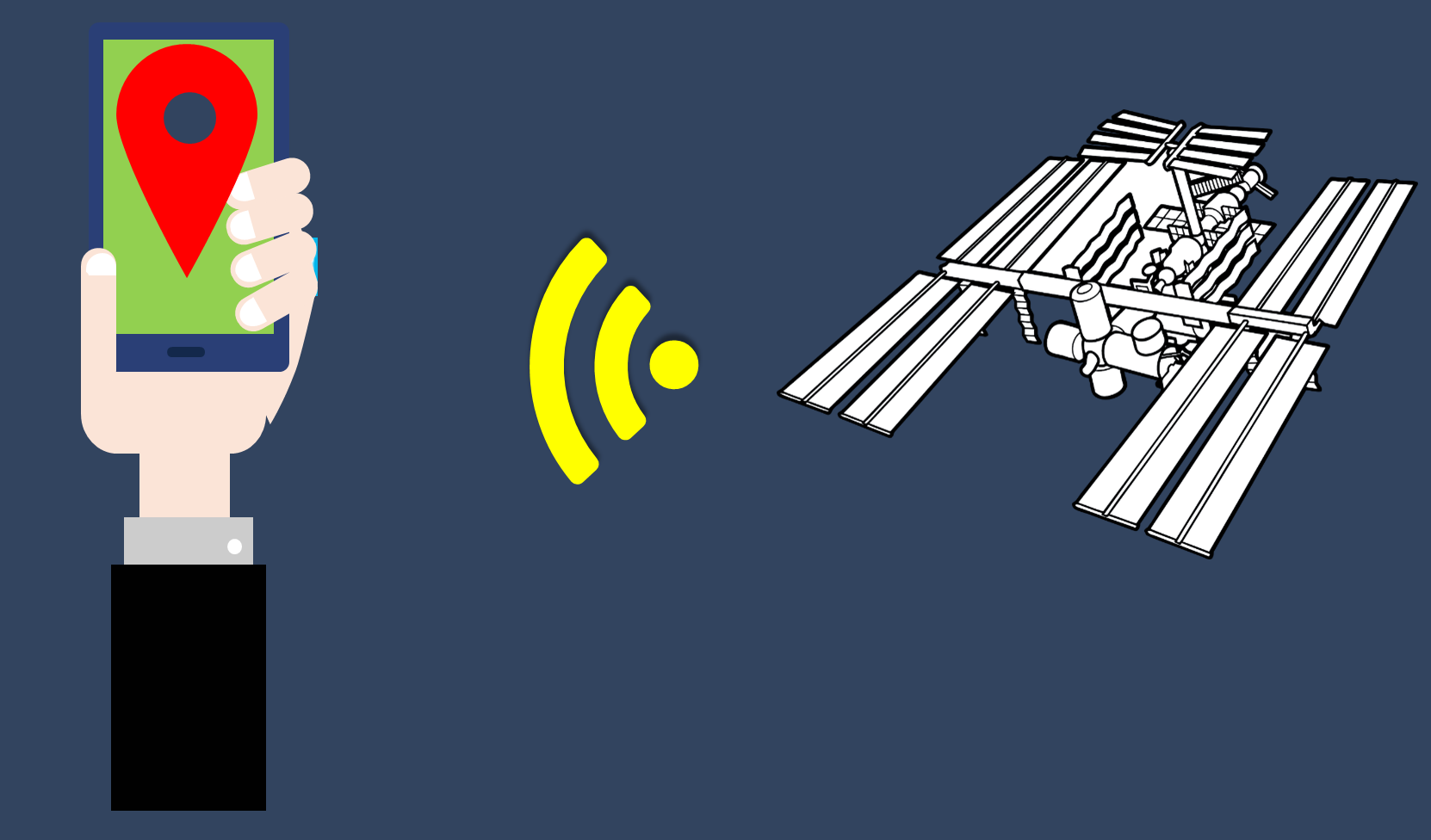
The next method is by connecting to a geolocation satellite. These values tend be extremely accurate, usually within 10 meters. The problem is you have to have an unobstructed view to one of the satellites, which is generally when you are outside.
This means when you are inside a building you cannot use this method, but the Wifi method should kick in instead. However, if either of these methods are unavailable and you have a cellular device the last option is to triangulate cell tower positions.
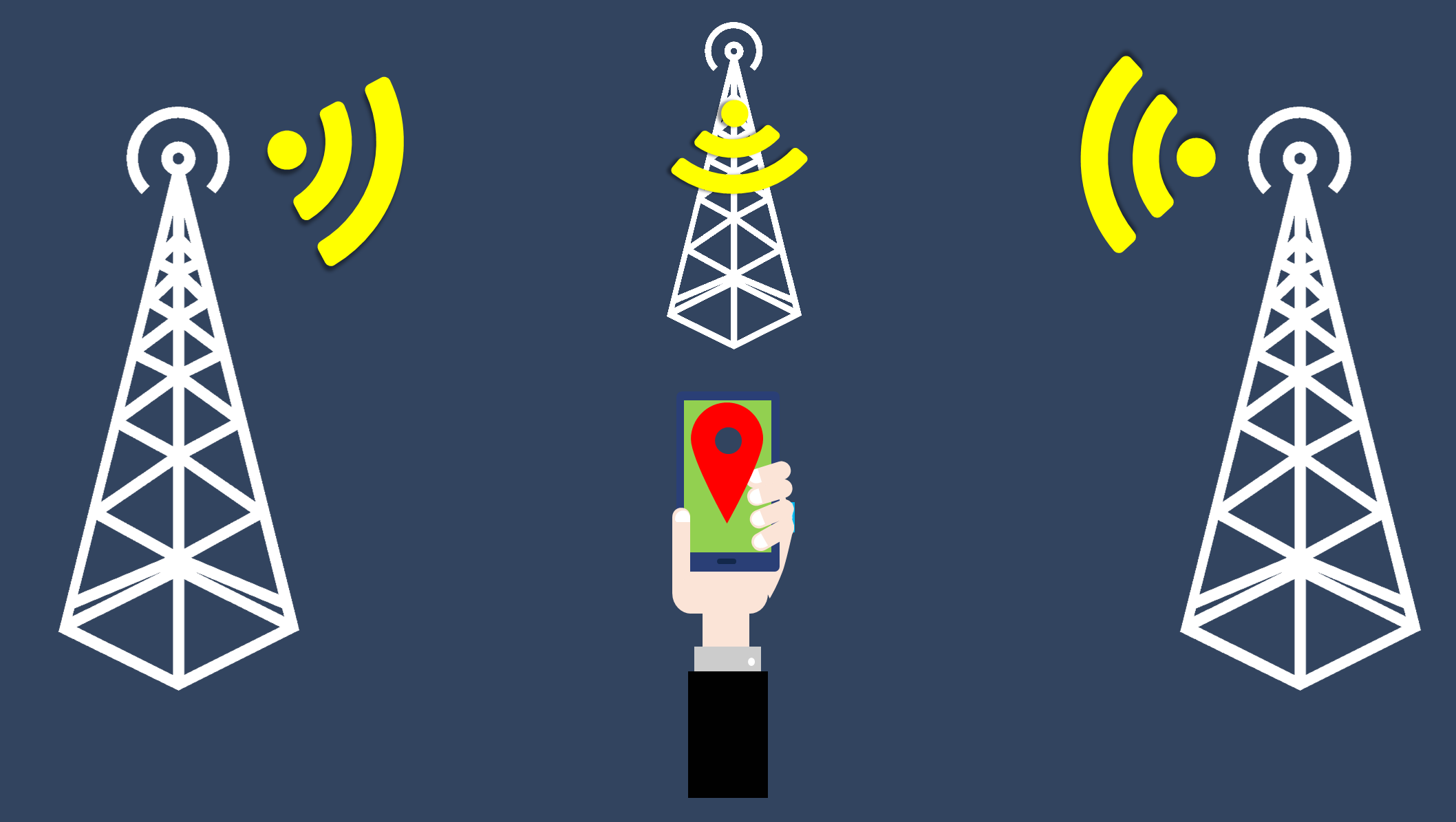
The accuracy from this technique is not very good, typically between 1000 and 3500 meters. Because these methods can vary it is a good idea to always check the accuracy to see if you need to adjust your application's response accordingly. Remember you do not control how the user’s device gets their coordinates, but you can determine how you use the position value based on accuracy.
iOS Approximate Location
Apple introduced a new setting in iOS 14, approximate location. The intent is to protect the user's privacy by providing a broad range of location. Since devices can provide highly accurate locations this could lead to bad guys finding you. The approximate location setting adds some obfuscation to your location. My interpretation is it will report your position similar to if it were triangulated off cell towers, or roughly within a 1km radius.
The problem this poses is when your application needs precise locations. For example a ride-share application. The driver needs to know where the passenger stands so they can pick them up. Because Apple has not surfaced an option for users to control this on an app by app level it is an all or nothing setting. If the user choses approximate location for their iPhone it affects all apps. There is no flag or value you application can check to see if the location is accurate or approximate either. You can use the accuracy property to determine how true the position is being reported. Even setting the Highprecision property to true does not change the value. The value is reported in 15 minute increments. So no updates if the user moves you will not know for 15 minutes.
Geolocation is one of the cooler, under utilized web platform APIs. While not all web applications can benefit from geolocation, but many can.
The API is fairly simple to implement, but you need to only use it when it offers real value. Over using the API can and will drain the visitor’s battery and consume CPU cycles.
Location is determined by the device, which will use one of three techniques to determine location. Accuracy is provided by the device so you will know how reliable the location data is.
You can get a single location value or use the API to track changes to the device location. So you can display area attractions or monitor a user’s path.

We use cookies to give you the best experience possible. By continuing, we'll assume you're cool with our cookie policy.
Install Love2Dev for quick, easy access from your homescreen or start menu.
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
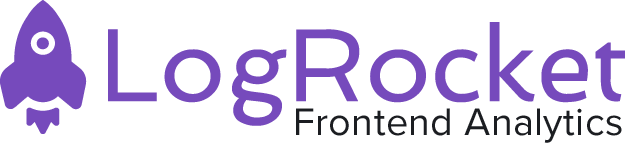
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
What you need to know while using the Geolocation API
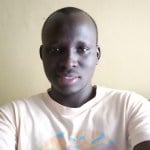
Introduction
The Geolocation API comes in handy when building an application that requires access to the precise client location. Among other reasons, you can use location services to provide a custom experience for your clients, or when building an application that requires real time location access. One such application is a fitness app that needs precise, real time location data for tracking a user’s route when taking a walk or jogging.
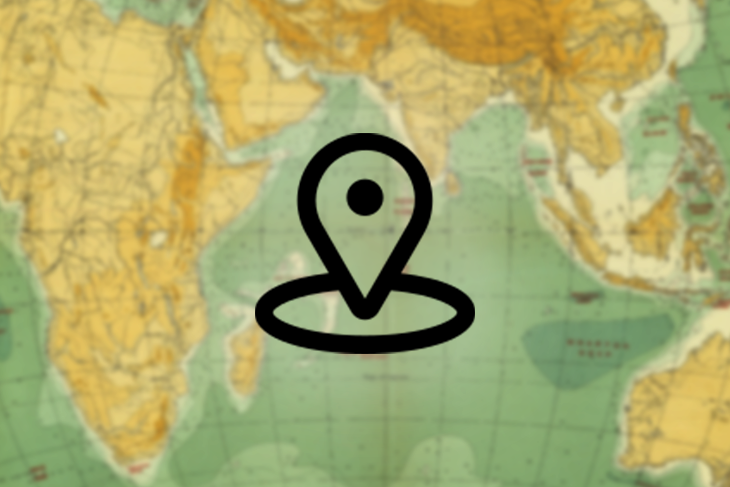
Because of privacy and safety concerns, the Geolocation API requires permission before you can access user location data. In this day and age, where an extreme state of paranoia is necessary to have some level of privacy and safety online, you should request access to location data gently and responsibly for users to grant permission. Additionally, having possession of such location data comes with greater ethical, moral, and legal responsibility.
Therefore, knowing how to use the Geolocation API only is insufficient. Using it effectively and responsibly is just as important.
The main goal of this article is to introduce you to the main features of the Geolocation API and explain how you can use it effectively. We shall also go above and beyond to highlight your responsibility as a developer when using the Geolocation API and explore the extent of browser support.
Let us start with a brief introduction to the Geolocation API in the section below.
What is the Geolocation API?
The Geolocation API is a built in API for accessing a client’s device location. Most recent versions of desktop browsers fully support this feature, but you cannot say the same about their mobile counterparts. You, therefore, need to have a fallback option if some browsers do not yet have full native support for this API.
The accuracy of the location data largely depends on the geolocation device used by the client’s device. The Geolocation API can retrieve location data from a GPS if a device has a GPS receiver. It can also infer user location from the IP address, WiFi, and Bluetooth MAC addresses, among others. The Geolocation API is agnostic of the technology the client’s device uses to access geolocation data.
There is no guarantee the geolocation information will be accurate, because this API can also use the IP address to infer user location in the absence of more accurate technologies, such as GPS receivers on the client’s device.
The browser exposes this API via the navigator.geolocation property. However, as already mentioned, you need to tread with caution, because browser support for this API is far from universal. You should only access it after checking for its availability; this will ensure you have a fallback option in case the client is using a browser that doesn’t support the API.
One fallback option is to use a third party geolocation service:
Before we proceed to the next section, you should note that most or all browsers expose the Geolocation API only if your site is using the secure HTTPS protocol.
Getting user location
Now let’s learn how to access user location using the Geolocation API and some of the key considerations while doing so.
First, we’ll see how you can use the getCurrentPosition method to access user location. The getCurrentPosition method takes up to three arguments.
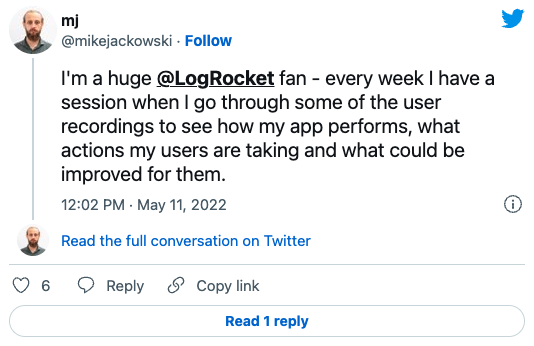
Over 200k developers use LogRocket to create better digital experiences

The first (and the only required) argument is a callback function invoked when the API receives the location data successfully. The API passes the device location data as an argument to the callback function.
The second is an optional callback function invoked when an error occurs, and the third argument is an object of options.
The code snippet below is an illustration of how you can use the getCurrentPosition method to access location data:
That is all it takes to access your client’s device location using the getCurrentPosition method of the Geolocation API.
However, there are a few best practices to know when using this method to access client location.
Access the Geolocation API in response to a user action
Do not attempt to access the Geolocation API immediately on page load. If you do, the API will instantly request the user for access to their location.
This provides for a poor user experience and will freak out some of your clients. Try to be extremely patient and gentle when asking for consent to get your clients to trust you with their location data.
Explain why you need location data
It is good practice to present the user with an explanation of why you need their location data and how you intend to use it before the browser displays a prompt with a “this site wants to know your location” message.
More great articles from LogRocket:
- Don't miss a moment with The Replay , a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
Additionally, you can also include a link to your privacy policy and terms of service.
Be explicit about the need for location data
Be explicit about the need for location data in your call to action directive. For example, if you have a button or link with the text “show stores near me,” you can also provide an accompanying message like, “this service requires access to your location” beside the button or as a tooltip.
Therefore, it won’t be surprising if the browser asks the user to grant access to their location.
Set a timeout in case the response takes too long
If a client is using a device with a GPS receiver like a mobile phone, the time it takes to access the location data, among other factors, depends on the atmospheric condition and location of the user. The GPS can take up to a minute or more to triangulate the device’s location.
You can decide what the acceptable waiting time is, and timeout the operation if it takes too long.
Furthermore, setting a timeout is equally useful if a user intentionally or unintentionally fails to respond to the permission request. You can then use an alternative third party geolocation service or show a nice message, like “The application took too long to access your location. Perhaps try again later.”
Handle errors gracefully
An error may occur, or a user can decline to grant access to location data. You can always pass another callback function as a second argument and use it to gracefully handle errors.
This is useful because browsers have features for disabling location lookup. If the user disables geolocation lookup in their browser or declines to grant permission, the second callback function passed to the getCurrentPosition method will be invoked with the error object. You can then take appropriate action thereafter.
Watching user location
Sometimes accessing geolocation data once may not be sufficient, especially when the user is on the move. You will have to use the watchPosition method to access location data whenever there is a change in the user’s location.
The watchPosition method is similar to the getCurrentPosition method. The first argument is a callback function invoked when the API receives location data, and it is also the only required argument. The second argument is also a callback function, but it is optional. It is invoked in case of an error. The third argument is an optional object.
Unlike the getCurrentPosition method, the watchPosition method returns a numeric ID that uniquely identifies the position watcher. You can use this ID to stop watching the user location with the clearWatch method.
The first callback function you pass to the watchPosition method is invoked multiple times to update client location if they are on the move or when more accurate geolocation data becomes available.
The code block below illustrates how you can use the watchPosition method. It is very similar to the getCurrentPosition method. The only difference is in the unique number ID the watchPosition method returns:
That is all there is to the watchPosition method of the Geolocation API. However, there are certain things you should take into consideration when using this method.
The goal of this article is to teach you how to use the Geolocation API in the best possible way. Therefore, all the considerations highlighted in the previous subsection also apply when using the watchPosition method.
Additionally, you should take note of the following:
Be mindful of efficiency
As highlighted above, watching user location necessitates multiple invocations of the watchPosition method. This, by all means, is a computationally expensive operation. It requires computing resources to perform.
With the widespread use of mobile devices, you need to be mindful of the impacts of repeatedly invoking the watchPosition method on your users in terms of performance. Similarly, a mobile device using a GPS receiver will listen for satellite signals when triangulating device location. Constantly watching user location can drain lots of battery power.
Only watch position if you must
As mentioned above, watching user locations can be an expensive operation. You should use the watchPosition method if you are sure doing so will improve the quality of service for your clients.
If you must watch user location, it is best to stop tracking if the geolocation information ceases to change. Make sure to stop watching when it is no longer necessary to watch user location. We shall highlight how to stop watching user locations in the next section.
How to stop watching user location
If you start watching user location, then you certainly will have to stop watching at some point.
You can use the clearWatch method to stop watching user location. The clearWatch method takes the unique watch ID returned by the watchPosition method as an argument:
The code snippet above is an illustration of how you can use the clearWatch method to stop watching user location.
Your responsibilities when using the Geolocation API
Now that you know what the Geolocation API is and how to use it, let us look at your responsibilities as a developer.
When using this API, you are essentially asking users to trust you with their location. Such sensitive data comes with lots of responsibilities on your part.
Highlighted below are some of these responsibilities. They mainly center around data protection and privacy policy. It may not necessarily be an exhaustive list, but it covers most of the basics.
Compliance with data protection laws
As you use the Geolocation API, it is your responsibility to comply with the relevant privacy and data protection laws within your jurisdiction. You should be aware that some data privacy and security legislation transcend territorial boundaries.
One fine example is the General Data Protection Regulation (GDPR) passed by the European Union. If your application is used by people in the EU, noncompliance with the GDPR will attract tough penalties irrespective of where you are in the world.
Terms of service and privacy policy
When you use the Geolocation API, you are asking users to grant access to sensitive data. Therefore, it is your responsibility to provide access to your terms of service and privacy policy. Providing terms of service and privacy policy will inform the users of the privacy implications of using the service and help them make informed decisions.
Probably not many will read the terms of service and privacy policy, but you will have done your part as a developer. You can also go ahead and present the, “by using this service, you agree to our terms of service and privacy policy” message before the service becomes available.
Use data for the intended purpose
You should be explicit in how you intend to use the geolocation data in your terms of service. You should also use the data for the specified purpose and discard it thereafter unless the user gave you express permission to do otherwise.
Allow users to delete their data
If you intend to persist the geolocation data in a database, you should give users the liberty and the means to delete or update the data if they so wish.
Protect user data and safeguard their privacy
The responsibility of safeguarding the privacy of your clients lies squarely on your shoulders. One possible safeguard is to encrypt the data. Data encryption is crucial, especially if you intend to save the data to a database or the user has granted you express permission to transmit the data to a third party.
Browser support for the Geolocation API
As already highlighted in the introduction section, some browsers, especially mobile browsers, have yet to implement some functionalities of the Geolocation API.
Support in mobile browsers notwithstanding, most recent versions of popular modern browsers support the Geolocation API. Your safest bet is to have a fallback option if your goal is to provide support for all browsers. One typical example of a fallback option is to use third-party geolocation services, which mostly rely on the less accurate IP addresses to infer geolocation data.
The image below shows the browser support for the Geolocation API according to caniuse .
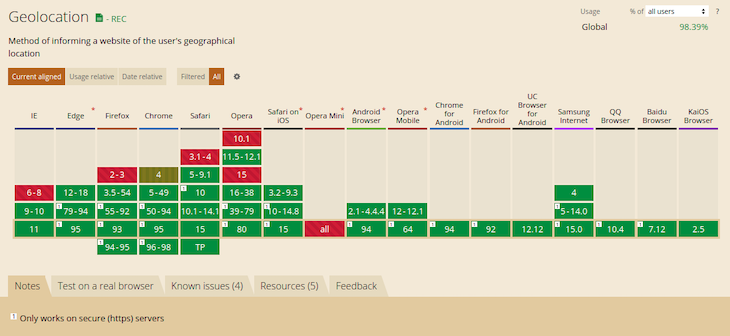
As mentioned in the subsections above, the Geolocation API will likely always be your first choice if you want to access your client’s location data in the browser. For privacy reasons, the browser needs user permission before granting your application access to user location data.
The location data is only as accurate as the geolocation technology the client’s device uses. The Geolocation API is agnostic of the underlying geolocation technology used by the device. It can use GPS, IP address, Bluetooth, and WiFi MAC addresses, among others, to infer user geolocation. Therefore the location may not always be accurate. Mobile devices with GPS receivers tend to provide more accuracy.
When using the Geolocation API, there are best practices that you need to follow. They will increase your chances of getting access to user location data and build confidence in your clients about their safety and privacy. It will also increase the possibility of adopting your product and services.
You are responsible for providing privacy and data protection after gaining access to the clients’ location data. Most countries have privacy and data protection legislation that may apply to you. Failure to comply with them will land you in trouble.
Hopefully, you enjoyed reading this article. Do you have more tips on how to effectively and responsibly use the Geolocation API? Let me know in the comments section below.
LogRocket : Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #vanilla javascript
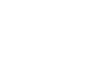
Stop guessing about your digital experience with LogRocket
Recent posts:.
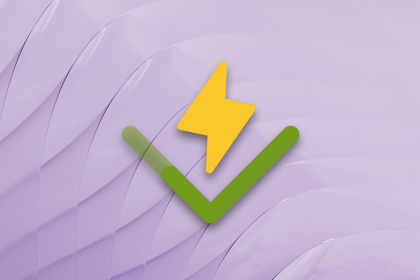
An advanced guide to Vitest testing and mocking
Use Vitest to write tests with practical examples and strategies, covering setting up workflows, mocking, and advanced testing techniques.
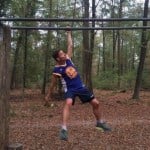
Optimizing rendering in Vue
This guide covers methods for enhancing rendering speed in Vue.js apps using functions and techniques like `v-once`, `v-for`, `v-if`, and `v-show`.
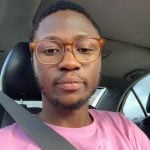
Using Google Magika to build an AI-powered file type detector
Magika offers extremely accurate file type identification, using deep learning to address the limitations of traditional methods.

Unistyles vs. Tamagui for cross-platform React Native styles
Unistyles and Tamagui both help address the challenges of creating consistent and responsive styles across different devices.
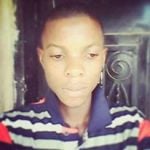
2 Replies to "What you need to know while using the Geolocation API"
You can use the IP geolocation API such as https://www.ip2location.com because it is less intrusive.
In the world, https://ipstack.com/ provides one of the top IP to geolocation APIs and global IP database services.
The geolocation object
Describing a position, handling errors, geolocation live example, prompting for permission, browser compatibility.
Secure context This feature is available only in secure contexts (HTTPS), in some or all supporting browsers .
The Geolocation API allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information.
The Geolocation API is published through the navigator.geolocation object.
If the object exists, geolocation services are available. You can test for the presence of geolocation thusly:
Note: On Firefox 24 and older versions, "geolocation" in navigator always returned true even if the API was disabled. This has been fixed with Firefox 25 to comply with the spec. ( bug 884921 ).
Getting the current position
To obtain the user's current location, you can call the getCurrentPosition() method. This initiates an asynchronous request to detect the user's position, and queries the positioning hardware to get up-to-date information. When the position is determined, the defined callback function is executed. You can optionally provide a second callback function to be executed if an error occurs. A third, optional, parameter is an options object where you can set the maximum age of the position returned, the time to wait for a request, and if you want high accuracy for the position.
Note: By default, getCurrentPosition() tries to answer as fast as possible with a low accuracy result. It is useful if you need a quick answer regardless of the accuracy. Devices with a GPS, for example, can take a minute or more to get a GPS fix, so less accurate data (IP location or wifi) may be returned to getCurrentPosition() .
The above example will cause the do_something() function to execute when the location is obtained.
Watching the current position
If the position data changes (either by device movement or if more accurate geo information arrives), you can set up a callback function that is called with that updated position information. This is done using the watchPosition() function, which has the same input parameters as getCurrentPosition() . The callback function is called multiple times, allowing the browser to either update your location as you move, or provide a more accurate location as different techniques are used to geolocate you. The error callback function, which is optional just as it is for getCurrentPosition() , can be called repeatedly.
Note: You can use watchPosition() without an initial getCurrentPosition() call.
The watchPosition() method returns an ID number that can be used to uniquely identify the requested position watcher; you use this value in tandem with the clearWatch() method to stop watching the user's location.
Fine tuning response
Both getCurrentPosition() and watchPosition() accept a success callback, an optional error callback, and an optional PositionOptions object.
A call to watchPosition could look like:
The user's location is described using a Position object referencing a Coordinates object.
The error callback function, if provided when calling getCurrentPosition() or watchPosition() , expects a PositionError object as its first parameter.
HTML Content
Javascript content, live result.
Any add-on hosted on addons.mozilla.org which makes use of geolocation data must explicitly request permission before doing so. The following function will request permission in a manner similar to the automatic prompt displayed for web pages. The user's response will be saved in the preference specified by the pref parameter, if applicable. The function provided in the callback parameter will be called with a boolean value indicating the user's response. If true , the add-on may access geolocation data.
Availability
As WiFi-based locationing is often provided by Google, the vanilla Geolocation API may be unavailable in China. You may use local third-party providers such as Baidu , Autonavi , or Tencent . These services use the user's IP address and/or a local app to provide enhanced positioning.
- navigator.geolocation
- Geolocation API on w3.org
- Who moved my geolocation? (Hacks blog)
Document Tags and Contributors
- Intermediate
- Coordinates
- Geolocation
- PositionError
- PositionOptions
- Navigator.geolocation
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.

IMAGES
VIDEO
COMMENTS
The Navigator object has a read-only Geolocation instance variable. You obtain location information from this Geolocation object. The parameters to the Geolocation methods that get location information are mostly callbacks, instances of PositionCallback or PositionErrorCallback. Because there may be a delay in getting location information, it ...
My objective is to return the coordinates of my geolocation using Safari on an iOS device. On my desktop browser, I am calling the function tryGeolocation() below with a Google Maps Geolocation A...
The Geolocation API allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information. WebExtensions that wish to use the Geolocation object must add the "geolocation" permission to their manifest. The user's operating system will prompt the user to allow location access the first time it is ...
Join the waiting lists. Using the Geolocation API we can ask the browser for the user's position coordinates. The browser exposes a navigator.geolocation object, through which we'll do all the geolocation operations. It's only available on pages served using HTTPS, for security purposes, and it's available on all modern browsers.
The Geolocation interface doesn't inherit any method.. Geolocation.getCurrentPosition() Determines the device's current location and gives back a GeolocationPosition object with the data.. Geolocation.watchPosition() Returns a long value representing the newly established callback function to be invoked whenever the device location changes.. Geolocation.clearWatch()
The Geolocation API is supported across all major internet browsers, including Chrome, Firefox, and Safari. Although, for security reasons, your site would need an SSL certificate to use this web API. # api # geolocation-api # web-api. Learn API Development tips & tricks.
Using the Geolocation API. Secure context: This feature is available only in secure contexts (HTTPS), in some or all supporting browsers. The Geolocation API is used to retrieve the user's location, so that it can for example be used to display their position using a mapping API. This article explains the basics of how to use it.
The geolocation API allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information. The geolocation object. The geolocation API is published through the navigator.geolocation object.. If the object exists, geolocation services are available.
HTML5 Geolocation in Safari 5. HTML5 Geolocation API lets you share your location with your favorite web sites. A JavaScript can capture your latitude and longitude, can be sent to backend web server, and do fancy location-aware things like finding local businesses or showing your location on a map.
Geolocation. - REC. Method of informing a website of the user's geographical location. Usage % of. Global. 98.2%. Current aligned. Usage relative. Date relative.
So, the API is safe to use. Using the geolocation API combined with a map service like Microsoft Bing Maps or Google Maps Platforms provide a rich end user experience. You can add a map service to your site to add more value and app like experiences to extend your brand. Now you can pinpoint where the visitor is located and add nearby locations ...
The Geolocation API is agnostic of the technology the client's device uses to access geolocation data. There is no guarantee the geolocation information will be accurate, because this API can also use the IP address to infer user location in the absence of more accurate technologies, such as GPS receivers on the client's device. ...
The Geolocation API allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information.
The Navigator.geolocation read-only property returns a Geolocation object that gives Web content access to the location of the device. This allows a website or app to offer customized results based on the user's location. Note: For security reasons, when a web page tries to access location information, the user is notified and asked to grant ...
I'm trying to get Safari to prompt me for my location with a very simple code. I'm working on a remote Macbook mini from my PC running Ubuntu. Inside the Macbook environment, the code below works on Firefox and Chrome perfectly fine. But on Safari nothing happens, I don't get prompt or nothing.
位置情報 API (Geolocation API) を使用すると、ユーザーが望む場合に、自分の位置情報をウェブアプリケーションに提供することができます。プライバシー上の理由から、ユーザーは位置情報を報告する許可を求められます。
timeout Optional. A positive long value representing the maximum length of time (in milliseconds) the device is allowed to take in order to return a position. The default value is Infinity, meaning that getCurrentPosition() won't return until the position is available. enableHighAccuracy Optional. A boolean value that indicates the application ...
2. I have a website that asks for geolocation. It should only ask the first time you open the website but I noticed that if you open the website on mobile (with Chrome or Safari) or on desktop with Safari, the website asks for permission every time you reload a page. If you open the website on a computer with Chrome it works as it should ...
In Firefox you can give the geolocation permission per website: I assume that it is the same for other browsers. So while the browser itself can have permission to access the Geolocation API, it could be blocked for your specific application. PS.: I wonder if Internet Explorer even supports the Geolocation API. For Safari, this support article ...
In the W3 example there is a clickable button that fires the call to GeoLocation when the user clicks it (which happens when the page has loaded). By setting a timeout, we allow Safari to deal with some other stuff (that might have something to do with calling the GeoLocation API for all I know) for a few (milli)seconds.