Web Animations in Safari 13.1
Apr 8, 2020
by Antoine Quint
With the release of iOS 13.4, iPadOS 13.4, and Safari 13.1 in macOS Catalina 10.15.4, web developers have a new API at their disposal: Web Animations . We have been working on this feature for well over 2 years and itâs now available to all Safari users, providing a great programmatic API to create and control animations using JavaScript.
In this article we will discuss the benefits of this new API, how best to detect its availability, how it integrates with existing features such as CSS Animations and CSS Transitions, and the road ahead for animation technology in WebKit.

A Little History
The WebKit team came up with original proposals for CSS Animations and CSS Transitions back in 2007 and announced them on this blog. Over the years these specifications have matured and become W3C standards and an integral part of the web platform.
With these technologies, integrating animations in web content became simple, removing the requirement for developers to write JavaScript while providing better performance and power consumption by allowing browsers to use hardware acceleration when available and integrate animations in the layout and rendering pipeline.
As a web developer, Iâve enjoyed the simplicity and great performance of CSS Animations and CSS Transitions. I believe it is those virtues that have allowed animations to become a powerful tool for web developers. However, in my day-to-day work, I also found a few areas where these technologies were frustrating: dynamic creation, playback control, and monitoring an animationâs lifecycle.
The great news is that these issues are all taken care of by the new Web Animations API. Letâs see how to leverage this new API to improve everyday code in these areas.
Part I â Animation Creation
While CSS allows you to very easily animate a state change (the appearance of a button, for instance) it will be a lot trickier if the start and end values of a given animation are not known ahead of time. Typically, web developers would deal with those cases with CSS Transitions:
While this may look like a reasonable amount of code, there are additional factors to consider. Forcing a style invalidation will not let the browser perform that task at the time it will judge most appropriate. And this is just one single animation; what if another part of the page, possibly even a completely different JavaScript library, also needed to create an animation? This would multiply forced style invalidations and degrade performance.
And if you consider using CSS Animations instead, you would have to first generate a dedicated @keyframes rule and insert it inside a <style> element, failing to encapsulate what is really a targeted style change for a single element and causing a costly style invalidation.
The value of the Web Animations API lies in having a JavaScript API that preserves the ability to let the browser engine do the heavy lifting of running animations efficiently while enabling more advanced control of your animations. Using the Web Animations API, we can rewrite the code above with a single method call using Element.animate() :
While this example is very simple, the single Element.animate() method is a veritable Swiss Army knife and can express much more advanced features. The first argument specifies CSS values while the second argument specifies the animationâs timing . We wonât go into all possible timing properties, but all of the features of CSS Animations can be expressed using the Web Animations API. For instance:
Now we know how to create an animation using the Web Animations API, but how is it better than the code snippet using CSS Transitions? Well, that code tells the browser what to animate and how to animate it, but does not specify when . Now the browser will be able to process all new animations at the next most opportune moment with no need to force a style invalidation. This means that animations you author yourself as well as animations that may originate from a third-party JavaScript library â or even in a different document (for instance, via an <iframe> ) â will all be started and progress in sync.
Part II â Playback Control
Another shortcoming with existing technologies was the lack of playback control: the ability to pause, resume, and seek animations and control their speed. While the animation-play-state property allows control of whether a CSS Animation is paused or playing, there is no equivalent for CSS Transitions and it only controls one aspect of playback control. If you want to set the current time of an animation, you can only resort to roundabout techniques such as clever manipulations of negative animation-delay values, and if you want to change the speed at which an animation plays, the only option is to manipulate the timing values.
With the Web Animations API, all these concerns are handled by dedicated API. For instance, we can manipulate playback state using the play() and pause() methods, query and set time using the read-write currentTime property, and control speed using playbackRate without modifying duration:
This gives developers control over the behavior of animations after they have been created. It is now trivial to perform tasks which would have been previously daunting. To toggle the playback state of an animation at the press of a button:
To connect the progress of an animation to an <input type="range"> element:
Thanks to the Web Animations API making playback control a core concept for animations, these simple tasks are trivial and more complex control over an animationâs state can be achieved.
Part III â Animation Lifecycle
While the transition* and animation* family of DOM events provide information about when CSS-originated animations start and end, it is difficult to use them correctly. Consider fading out an element prior to removing it from the DOM. Typically, this would be written this way using CSS Animations:
Seems correct, but on further inspection there are problems. This code will remove the element as soon as an animationend event is dispatch on the element, but since animation events bubble, the event could come from an animation completing in a child element in the DOM hierarchy, and the animations could even be named the same way. There are measures you can take to make this kind of code safer, but using the Web Animations API, writing this kind of code is not just easier but safer because you have a direct reference to an Animation object rather than working through animation events scoped to an elementâs hierarchy. And on top of this, the Web Animations API uses promises to monitor the ready and finished state of animations:
Consider how complex the same task would have been if you wanted to monitor the completion of a number of CSS Animations targeting several elements prior to removing a shared container. With the Web Animations API and its support for promises this is now expressed concisely:
Integration with CSS
Web Animations are not designed to replace existing technologies but rather to tightly integrate with them. You are free to use whichever technology you feel fits your use case and preferences best.
The Web Animations specification does not just define an API but also aims to provide a shared model for animations on the web; other specifications dealing with animations are defined with the same model and terminology. As such, itâs best to understand Web Animations as the foundation for animations on the web, and think of its API as well as CSS Transitions and CSS Animations as layers above that shared foundation.
What does this mean in practice?
To make a great implementation of the Web Animations API, we had to start off fresh with a brand new and shared animation engine for CSS Animations, CSS Transitions, and the new Web Animations API. Even if you donât use the Web Animations API, the CSS Animations and CSS Transitions youâve authored are now running in the new Web Animations engine. No matter which technology you choose, the animations will all run and update in sync, events dispatched by CSS-originated animation and the Web Animations API will be delivered together, etc.
But what may matter even more to authors is that the entire Web Animations API is available to query and control CSS-originated animations! You can specify animations in pure CSS but also control them with the Web Animations APIs using Document.getAnimations() and Element.getAnimations() . You can pause all animations running for a given document this way:
What about SVG? At this stage, SVG Animations remain distinct from the Web Animations model, and there is no integration between the Web Animations API and SVG. This remains an area of improvement for the Web platform.
Feature Detection
But before you start adopting this new technology in your projects, there are some further practical considerations that you need to be aware of.
Since this is new technology, it is important to use feature detection as users gradually update their browsers to newer versions with support for Web Animations. Detecting the availability of the various Web Animations API is simple. Here is one correct way to detect the availability of Element.animate() :
While Safari is shipping the entire Web Animations API as a whole, other browsers, such as Firefox and Chrome, have been shipping Element.animate() for a long time already, so itâs critical to test individual features separately. So, if you want to use Document.getAnimations() to query all running animations for a given document , make sure to feature detect that featureâs availability. As such the snippet further above would be better written this way:
There are parts of the API that arenât yet implemented in Safari. Notably, effect composition is not supported yet. Before trying to set the composite property when defining the timing of your animation, you can check whether it is supported this way:
Animations in Web Inspector
Also new in the latest Safari release: CSS Animations and CSS Transitions can be seen in Web Inspector in the new Media & Animations timeline in the Timelines Tab, organized by animation-name or transition-property properties for each element target. When used alongside other timelines, it can be helpful to correlate how that particular CSS Animation or CSS Transition was created, such as by looking at script entries in the JavaScript & Events timeline.
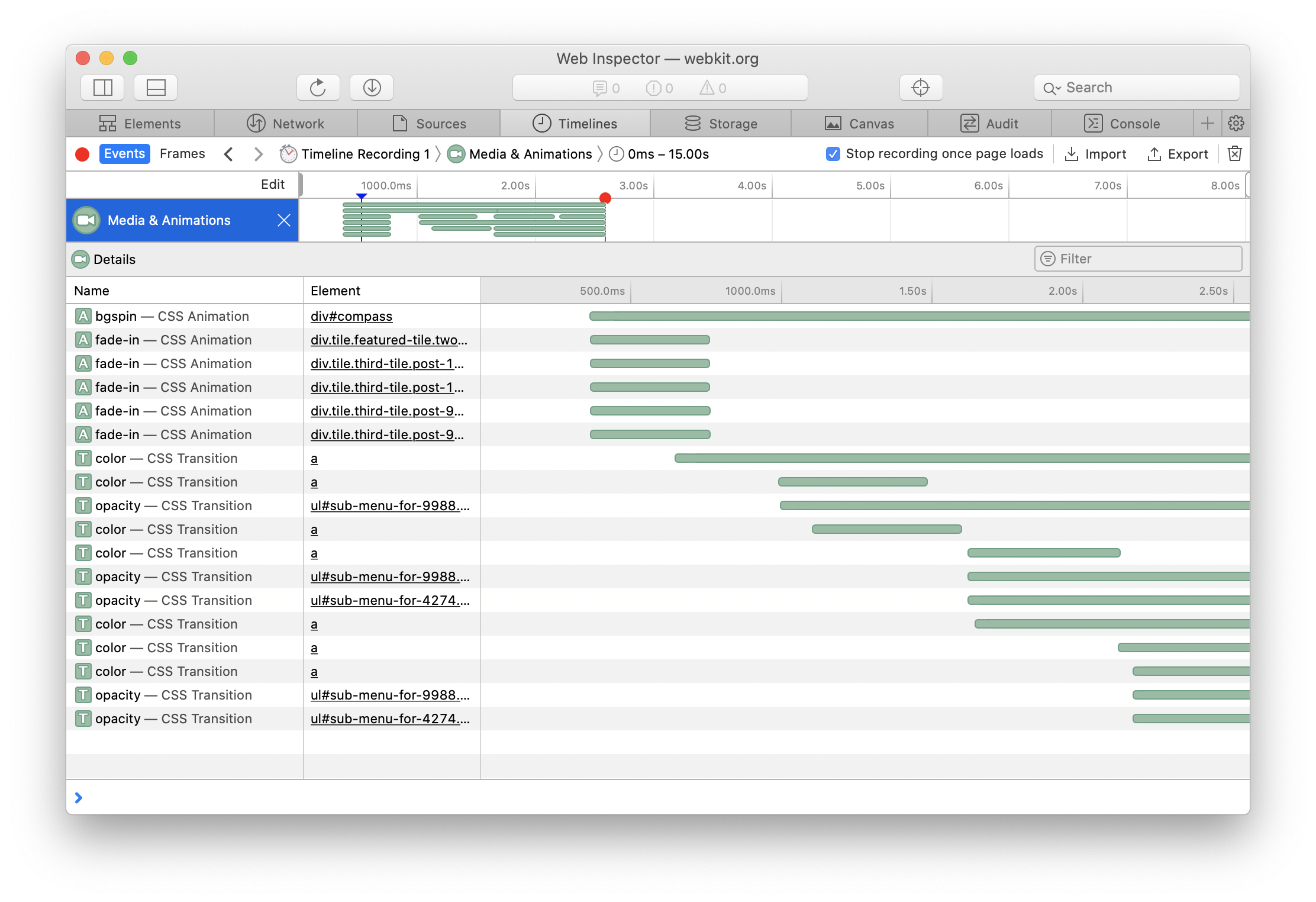
Starting in Safari Technology Preview 100 , Web Inspector shows all animations, whether they are created by CSS or using the JavaScript API, in the Graphics Tab. It visualizes each individual animation object with lines for delays and curves for keyframes, and provides an in-depth view of exactly what the animation will do, as well as information about how it was created and some useful actions, such as logging the animation object in the Console. These are the first examples of what Web Animations allow to improve Web Inspector for working with animations, and weâre looking forward to improving our tools further.
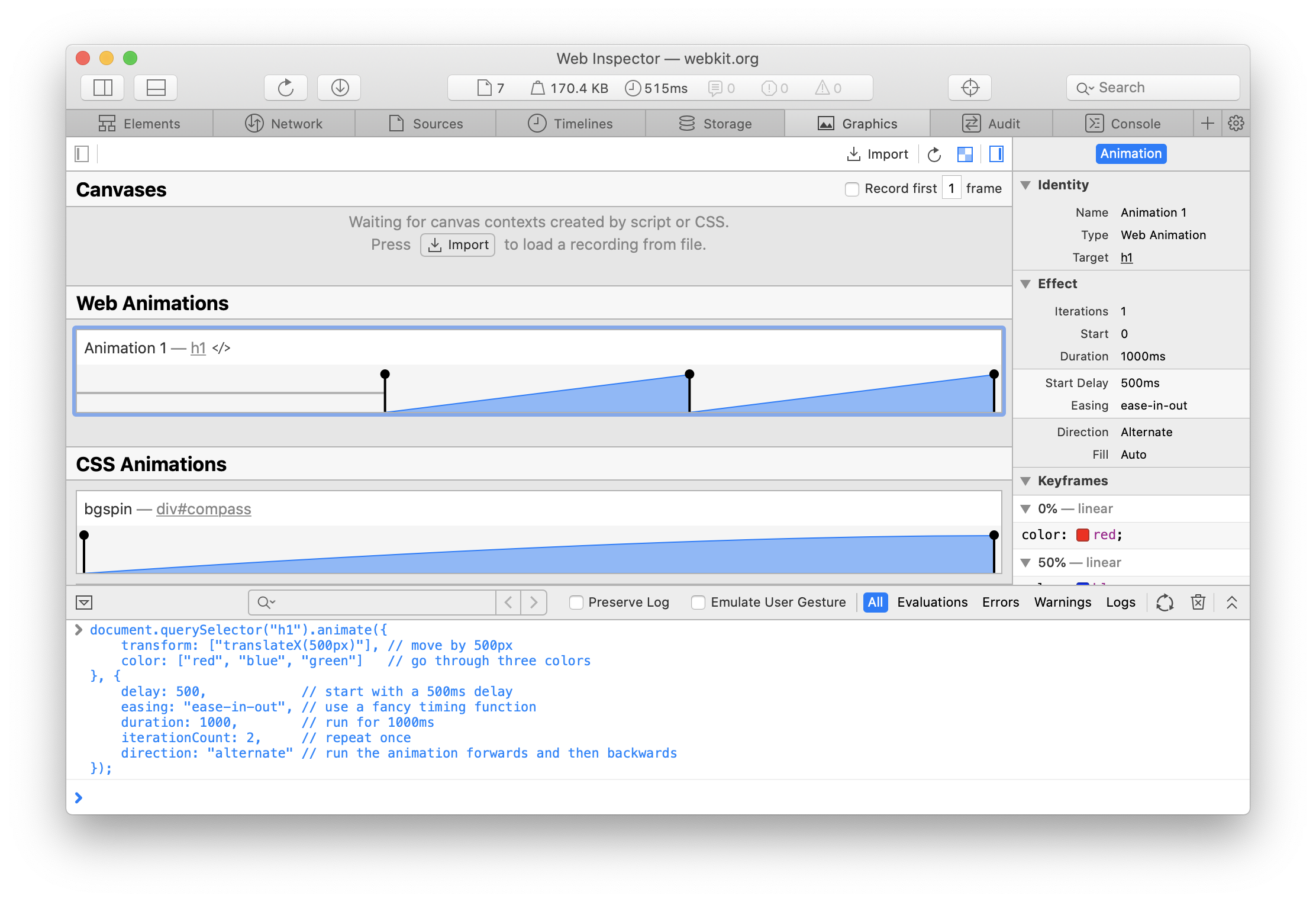
The Road Ahead
Shipping support for Web Animations is an important milestone for animations in WebKit. Our new animation engine provides a more spec-compliant and forward-looking codebase to improve on. This is where developers like you come in: itâs important we hear about any compatibility woes you may run into with existing content using CSS Animations and CSS Transitions, but also when adopting the new Web Animations API in your content.
The transition to the new Web Animations engine allowed us to address known regressions and led to numerous progressions with Web Platform Tests, improving cross-browser compatibility. If you find your animations running differently in Safari, please file a bug report on bugs.webkit.org so that we can diagnose the issue and establish if it is an intentional change in behavior or a regression that we should address.
Weâre already working on improving on this initial release and you can keep an eye out for future improvements by monitoring this blog and the release notes for each new Safari Technology Preview release .
You can also send a tweet to @webkit or @jonathandavis to share your thoughts on our new support for Web Animations.
Safari CSS Visual Effects Guide
- Table of Contents
- Jump To
- Download Sample Code
Animating CSS Transitions
You can create animations entirely in CSS, with no need for plug-ins, graphics libraries, or elaborate JavaScript programs. Normally, when the value of a CSS property changes, the affected elements are re-rendered immediately using the new property value. If you set CSS transition properties, however, any changes in the values of the specified CSS properties are automatically rendered as animations. This kind of automatic animation is called a transition .
You trigger a transition simply by changing any of the specified CSS values. CSS property values can be changed by a pseudoclass, such as :hover , or by using JavaScript to change an elementâs class name or to change its individual CSS properties explicitly. The animation flows smoothly from the original state to the changed state using a transition timing function over a specified duration.
For more complex animations that can use arbitrary intermediate states and trigger conditions, see Animating With Keyframes .
Transitions are especially powerful if you combine them with 2D and 3D transforms. For example, Figure 5-1 shows the results of applying an animated transition to an element as it rotates in 3D. See the CardFlip sample code project for the complete source code for this example.
Animated transitions are a W3C draft specification: http://www.w3.org/TR/css3-transitions/ .
Setting Transition Properties
To create an animated transition, first specify which CSS properties should be animated, using the -webkit-transition-property property, a CSS property that takes other CSS properties as arguments. Set the duration of the animation using the -webkit-transition-duration property.
For example, Listing 5-1 creates a div element that fades out slowly over 2 seconds when clicked.
Listing 5-1 Setting transition properties
There are two special transition properties: all and none :
-webkit-transition-property: all;
-webkit-transition-property: none;
Setting the transition property to all causes all changes in CSS properties to be animated for that element. Setting the transition property to none cancels transition animation effects for that element.
To set up an animation for multiple properties, pass multiple comma-separated parameters to -webkit-transition-property and -webkit-transition-duration . The order of the parameters determines which transition the settings apply to. For example, Listing 5-2 defines a two-second -background-color transition and a four-second opacity transition.
Listing 5-2 Creating multiple transitions at once
Using Timing Functions
Timing functions allow a transition to change speed over its duration. Set a timing function using the -webkit-transition-timing-function property. Choose one of the prebuilt timing functionsâ ease , ease-in , ease-out , or ease-in-out âor specify cubic-bezier and pass in control parameters to create your own timing function. For example, the following snippet defines a 1-second transition when opacity changes, using the ease-in timing function, which starts slowly and then speeds up:
Using the cubic-bezier timing function, you can, for example, define a timing function that starts out slowly, speeds up, and slows down at the end. The timing function is specified using a cubic Bezier curve, which is defined by four control points, as Figure 5-2 illustrates. The first and last control points are always set to (0,0) and (1,1), so you specify the two in-between control points. The points are specified using x,y coordinates, with x expressed as a fraction of the overall duration and y expressed as a fraction of the overall change.
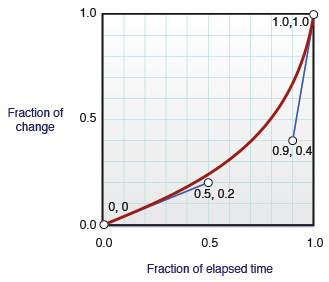
For example, Listing 5-3 creates a 2-second animation when the opacity property changes, using a custom Bezier path.
Listing 5-3 Defining a custom timing function
In the example just given, the custom timing function starts very slowly, completing only 20% of the change after 50% of the duration, and 40% of the change after 90% of the duration. The animation then finishes swiftly, completing the remaining 60% of the change in the remaining 10% of the duration.
Delaying the Start
By default, a transition animation begins as soon as one of the specified CSS properties changes. To specify a delay between the time a transition property is changed and the time the animation begins, use the -webkit-transition-delay property. For example, the following snippet defines an animation that beings 100ms after a property changes:

Setting Several Transition Properties At Once
You can set an animationâs transition properties, duration, timing function, and delay using a single shorthand property: -webkit-transition . For example, the following snippet uses the :HOVER pseudostyle to cause img elements to fade in and out when hovered over, using a one-second animation that begins 100 ms after the hover begins or ends, and using the ease-out timing function:
Handling Intermediate States and Events
When applying a transition to an elementâs property, the change animates smoothly from the old value to the new value and the property values are recomputed over time. Consequently, getting the value of a property during a transition may return an intermediate value that is the current animated value, not the old or new value.
For example, suppose you define a transition for the left and background-color properties and then set both property values of an element at the same time. The elementâs old position and color transition to the new position and color over time as illustrated in Figure 5-3 . Querying the properties in the middle of the transition returns an intermediate location and color for the element.
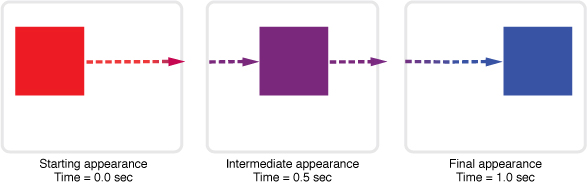
To determine when a transition completes, set a JavaScript event listener function for the DOM event that is sent at the end of a transition. The event is an instance of WebKitTransitionEvent , and its type is webkitTransitionEnd .
For example, the snippet in Listing 5-4 displays an alert panel whenever a transition ends.
Listing 5-4 Detecting transition end events
Copyright © 2016 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2016-10-27
Sending feedback…
We’re sorry, an error has occurred..
Please try submitting your feedback later.
Thank you for providing feedback!
Your input helps improve our developer documentation.
How helpful is this document?
How can we improve this document.
* Required information
To submit a product bug or enhancement request, please visit the Bug Reporter page.
Please read Apple's Unsolicited Idea Submission Policy before you send us your feedback.
Adobe Community
- Global community
- æ„æŹèȘăłăă„ăă㣠Dedicated community for Japanese speakers
- íê” ì»€ëź€ëí° Dedicated community for Korean speakers
- Premiere Pro
- Discussions
Issue with opacity (transparency) transition
Copy link to clipboard
1 Correct answer
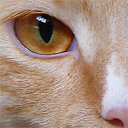
never-displayed
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . Weâll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[UI] CSS animations and transitions below 60fps become progressively slower #6302
Retroeer commented Aug 24, 2024
No branches or pull requests
Cross Browser Opacity
These days, you really don’t have to worry about opacity being a difficult thing cross-browser. You just use the opacity property, like this:
0 is totally transparent (will not be visible at all, like visibility: hidden;) and 1 is totally opaque (default). Anything in between is partially transparent.
For historical reasons, this is how is we used to do it:
Actually missed the IE8 opacity brouhaha. Thanks for including that!
Yep. I didnât know about that one either. The only issue I have with this snippet is that it’s not valid CSS.
Nevertheless, I’m gonna use it.
add display:inline-block will fix that. xD
Che Guevara Killer!
@Amr SubZero I created an account only to thank you, this fixes the issue of IE where an element was not passing opacity down to its children.
Usefull :) Thanks!
attention – sequence
.yourselector { background:#000; opacity: .75; /* standard: ff gt 1.5, opera, safari */ -ms-filter: âalpha(opacity=75)â; /* ie 8 */ filter: alpha(opacity=75); /* ie lt 7 */ -khtml-opacity: .75; /* safari 1.x */ -moz-opacity: .75; /* ff lt 1.5, netscape */ }
If you want opacity to also work in IE8’s emulating IE7 mode, the order should be:
-ms-filter:”progid:DXImageTransform.Microsoft.Alpha(Opacity=50)”; // first filter: alpha(opacity=50); // second
If you donât use this order, IE8 emulating IE7 doesnât apply the opacity, although IE8 and IE7 native do.
What about keeping the text inside the layer normal?
yeah! I’m wondering how to do this too..
If you set a parent container to an opacity less than 100%, all of its children will also be less than 100%, not because it cascades, but because the parent is dimmed. If the parent is dimmed to 75%, even if you give the child 100% opacity, it will only be 100% of 75%, which is 75%.
What I do is just use a semi-transparent PNG as a background image for the parent, and then the children can have full opacity.
inb4 IE6 doesn’t render 24bit pngs… Eff ie6, I’m done pandering to ie6, and enabling everyone who still uses it. The use of a semi transparent png is not going to break the site, but it will certainly affect the prettyness, which is all the more reason for people to upgrade.
You need to prevent the style from cascading… you need to wrap your text in an element with an opacity of 100.
I was wrong: https://css-tricks.com/non-transparent-elements-inside-transparent-elements/
I find that I use this snippet more than any other on the site. Thanks! =D
Is this ok for IE9?
ie9 support “opacity: 0.5;” like chrome and firefox
thanks for update coded^^
Awesome “Submit Comment From”. Congratulations, the best i have ever find surfing in the internet world for years…
But I have one question… Why all of you “webmasters”, keep asking email , hum??? If i want an answer I just put my email or even better I contact you. If not I will just SIMPLY LIE! And use one like this one: [email protected] Take off this ârequired fieldsâ. I hate this mass standardization and globalization, it’s so kitsch and so predictable, is a false sentence to evoke a mystified concept from it.
Webmasters ask for emails to help identify spam, as well as uniquely identify users. If you don’t want to enter your email, then have faith that your posts will be removed as spam, and that you really can’t contribute, because you’re not anything more than a few “asdf”s
I LOLD when i read the “good browsers” thing
That my man is because you are an idiot
good information, very helpful. Thanks..
@asdasdasd: you should view this awesome submit form in IE8.
I tryed this on a PNG but then it get a black bar arround it.
Thanks this is a big help!
As Chris wrote here: https://css-tricks.com/64-css-transparency-settings-for-all-broswers/
Use the following anno 2011:
All my text is invisible because it is supposed to be completely transparent against a semi-opaque background… but I can’t get the opacity to work in IE 7/8. It’s nested inside a P that’s it.
I tried zoom:1 & Hilbrand’s fix for opacity and I still can’t get it to work. Am I doing something wrong or anyone know a code snippet to fix it?
Had to laugh at the reference to ‘Good Browsers’ – I’m glad things like this don’t need to be a long article as all the answers are in your snippet, which is great. :)
Thanks this has saved me hours!
Good Browsers you got that one right!
“itâs so kitsch and so predictable, is a false sentence to evoke a mystified concept from it” You should have been a writer! Haha.
The /* Good browsers */ comment made me laugh!
Thanks for the post, I use this snippet of code all the time when designing :)
Just have to say thanks for this. I continually refer to it!
This works great for me except for IE6 & IE7. It turns out that you need to capitalize the “A” and “O” like this:
filter: Alpha(Opacity=50);
my mistake! my last post was incorrect. using lower case works correctly in IE6 & IE7
filter: alpha(opacity=50);
Thanks again!
-ms-filter:âprogid:DXImageTransform.Microsoft.Alpha(Opacity=50)â;
This not works in IE8
What i have to do work it in IE8 & 7
I have used css pie for rounded corner but one problem is that for opacity i have used the above code but i can’t see the opacity.
if i remove csspie behavior then i see the opacity in IE8.
so is it problematic to include csspie? i dont find any solution pls help.
Have you created all these snippets as wordpress’ dynamic pages unlike wordpress posts?
No.. actually i have used this snippets in my html page and css.
Hi every time I need to design a css style, I come here. thanks a lot Chris
how bout -webkit-opacity? Is it for google chrome?
Any update on this?
The is an issue with using the opacity property and images:hover. This causes the images to “jump” a pixel for a strange reason. however the following fix works (I have no idea why):
box-shadow: #000 0em 0em 0em;
for more information: http://stackoverflow.com/questions/9581071/jquery-fading-images-with-html-resize-makes-them-move-sometimes
Good Job Mr.
Helps me a lot specially in IE issues
there is a better way found:
background-color: transparent; background-color: rgba(0,0,0,0.3); filter:progid:DXImageTransform.Microsoft.gradient(startColorstr=#30000000,endColorstr=#30000000); -ms-filter: “progid:DXImageTransform.Microsoft.gradient(startColorstr=#30000000,endColorstr=#30000000)”;
how to measure background size in IE8 when ms-filter not worked
sorry wrong post, that’s for transparent background only, but works without isolating ie7 8 files
Unfortunately I’m facing black border around button in IE7 while using opacity.
does any one have solution for this ?
Thanks, perfect :D
Thanks a million Chris. This helped me a lot :)
For IE7 you really to ensure it has a few of these as well… position: relative; z-index: 99; zoom: 1; overflow: visible;
Thanks. it was useful.
was really helpful
Hmm. This only seems to work when I drop the quotes around each filter.
Hi, IE9 compatibility mode the opacity not working. Is there any specific code for that…
Why would you use compatibility mode in IE9? Simple users don’t use that. Test on real IE8 if you want IE8 compatibility.
still laughing at the comment /* Good browsers */
. . . but that’s the truth :)
This code is not working on IE8. Anyone tell me the perfect solution. thanks
https://developer.mozilla.org/en-US/docs/Web/CSS/opacity “Firefox 3.5 and later do not support -moz-opacity”
The best place to find css tricks. IE8?! what were they thinking, MS, seriously?
Thanks for this! Gotta love still supporting IE8! ;)
need a solution for ie8 :|
Can we get this page updated or off the internet, please? This shit is ancient.
I’ll update it to be more clear and suggest the most modern simplest way.
Hi Chris, you put filter: alpha(opacity=50); under IE 5-7, but it works just fine in IE8, no need for that complicated line of code with DirectX… for IE8.
Am I missing something? It’s true I am testing this on IE11 emulating IE8.
Stop supporting legacy browsers.
Make a huge notification window when someone is trying to connect to the website through one of them, notifying him that he’s using a dinosaur-old web browser and that he should update. Even Microsoft is trying to get rid of IE!
It’s so ridiculous for IE 8, not support opacity, even filter:alpha(opacity=56). And why must use -ms-filter:”progid:DXImageTransform…bla.bla.bla”?
Silviu is correct… filter: alpha(opacity=50); works fine on IE8
Opacity is working fine in IE11 and Chrome but fails in IE11 – ENTERPRISE MODE. Kindly advice if any body had tried opacity for IE in enterprise mode and the issue is the background is completely grey in color which works fine in other browsers. have used {opacity: .3; filter: Alpha(Opacity=30)}. Kindly share a solution.
what is the use for
/* Safari 1.x */ -khtml-opacity: 0.5;
Hey there! That’s the the required prefix if you want to Safari version 1 to support opacity. Safari didn’t support that feature until later, so getting to work on that version will need that prefix on the property.
okey, thank you sir Geoff Graham
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Copy and paste this code: micuno *
Leave this field empty

IMAGES
VIDEO
COMMENTS
6. I have a bug on a opacity transition in both Chrome and Safari. When I hover over a parent div that triggers a opacity transition from 0 to 1 on a child div, content beneath the child div jitters for a split moment. I've tried using -webkit-font-smoothing: antialiased; on the body, but the effect still occurs.
Fuck Safari so much. I hate that devilspawn of a browser. My Safari (iOs 15.4.1) has troubles displaying CSS transitions with transform and opacity when different transition times are given for the two, like transition: opacity 400ms, transform 600ms. Just wanted to let you know, in case you encounter a similar situation in your work.
It seems Safari has a bug and chokes on transition: all; (or just transition: Xs; because 'all' is the default property). It even crashes some versions of desktop and iOS Safari. SOLUTION: Change it to transition: color 0.5s ease-in-out; (Or, of course any other property, just make sure it's not 'all'.) It could also be a problem specifically ...
I have a strange issue when using the latest Safari version (15.4) under Mac OS Monterey 12.3. If I use x-transition.opacity.duration.300ms or x-transition.opacity in general I get a flash frame after transition ends. It works absolutely fine on every other browser, and I am 100% sure it also worked on Safari < 15.4
I am trying to create a transition on a full screen overlay that is full width and full height with nonactive styles of visibility: hidden and opacity: 0.When clicking on a hamburger icon, an .active class is added to the div and it has the following styles: visibility: visible and opacity: 1.. Here is the CSS:
I have a strange issue when using the latest Safari version (15.4) under Mac OS Monterey 12.3. If I use x-transition.opacity.duration.300ms or x-transition.opacity in general I get a flash frame after transition ends. It works absolutely fine on every other browser, and I am 100% sure it also worked on Safari before I update.
When the button below is clicked, the next image will be given the highest z-index and its opacity will be faded from 0 to 1 over .5s. This process will cycle for each image. ... In iOS Safari, the transition of images is delayed. Discounting the 300ms delay for tapped elements on touch devices, an image still takes a lot longer than 300ms to ...
Instead of changing the opacity of the div (.sq) you need to change the opacity of the img that is the child of that div. Placing the opacity and transition on img.ws worked for me. Oh, and this is crazy simple when it is working, no need for jQuery at all in my opinion. August 13, 2012 at 2:38 pm #107863. DoPeT.
Is this not the proper why to right the transition rule for Safari? -webkit-transition: transform 0.3s ease 0s, opacity 0.2s ease 0s; /* Chrome 1-25, Safari 3.2+ */. To be honest I have no idea what the Codepen is supposed to be doing. It looks broken to me, what with unlinked images, displaced/disjointed text etc.
With the release of iOS 13.4, iPadOS 13.4, and Safari 13.1 in macOS Catalina 10.15.4, web developers have a new API at their disposal: and it's now available to all Safari users, providing a great programmatic API to create and control animations using JavaScript. In this article we will discuss the benefits of this new API, how best to ...
We faced an issue, where the node content was rendered to coordinates 0,0 (top-left corner) of the parent SVG. This is due to a bug in Safari, which affects foreignobjects to calculate the rendering position based on the top SVG and not the foreignobject itself.
Seems that there is an issue on Safari. The divs that have the animation set on them appear to have a more saturated background than the rest of the page. They actually shouldn't have any background so the opacity transition should apply only to text, right? Explicitly setting background to transparent doesn't solve the issue.
The Grow transition flickers toward the end of the transition on Safari (tested on macOS and iOS). The length of the flicker depends on the transition's duration. The transition is also not fading out properly. This appears to happen on any component that uses Grow (Dialogs, Snackbars, Menus, etc.) GIF from docs in Safari on macOS:
Setting Transition Properties. To create an animated transition, first specify which CSS properties should be animated, using the -webkit-transition-property property, a CSS property that takes other CSS properties as arguments. Set the duration of the animation using the -webkit-transition-duration property.. For example, Listing 5-1 creates a div element that fades out slowly over 2 seconds ...
border-radius with a value greater than zero. border-color transprent. opacity use on hover. The bug is present with regular CSS in Tailwind CSS context but when I remove completely Tailwind CSS regular CSS works well so it seems to be related with Tailwind CSS. I think next step is to look at Tailwind CSS base and reset đ”ïžââïž.
Solved: There seem to be an issue with the opacity transitions. I have the latest version and reseted my settings. I tried many different pictures but the - 14332357. Adobe Community. cancel. Turn on suggestions. Auto-suggest helps you quickly narrow down your search results by suggesting possible matches as you type. ...
Both are running on next 13 and using MUI 5. For us, it also happens when a route transition is triggered while a backdrop is visible. This happens while a dialog is open or our menu is open on which we add a backdrop. I'm glad someone found the root of the problem, as it was impossible for us to recreate the bug consistently.
Describe the bug. Animations and transitions are slower below 60fps, this means if you try to time something with these, it looking nice is entirely dependent on if your player's computer is ass or not. To Reproduce. Create a basic opacity: 0 to opacity: 1 animation (or transition, both get fucked) Cap your framerate to either 30 or 10
Cross Browser Opacity. Chris Coyier on Dec 2, 2014. These days, you really don't have to worry about opacity being a difficult thing cross-browser. You just use the opacity property, like this: .thing { opacity: 0.5; } 0 is totally transparent (will not be visible at all, like visibility: hidden;) and 1 is totally opaque (default).